stalker.models.shot.Shot¶
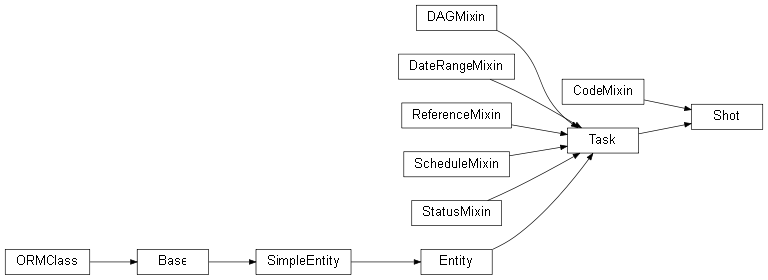
-
class
stalker.models.shot.
Shot
(code=None, project=None, sequences=None, scenes=None, cut_in=None, cut_out=None, source_in=None, source_out=None, record_in=None, image_format=None, fps=None, **kwargs)[source]¶ Bases:
stalker.models.task.Task
,stalker.models.mixins.CodeMixin
Manages Shot related data.
Warning
Deprecated since version 0.1.2.
Because most of the shots in different projects may going to have the same name, which is a kind of a code like SH001, SH012A etc., and in Stalker you can not have two entities with the same name if their types are also matching, to guarantee all the shots are going to have different names the
name
attribute of the Shot instances are automatically set to a randomly generated uuid4 sequence.Note
New in version 0.1.2.
The name of the shot can be freely set without worrying about clashing names.
Note
New in version 0.2.0.
Shot instances now can have their own image format. So you can set up different resolutions per shot.
Note
New in version 0.2.0.
Shot instances can now be created with a Project instance only, without needing a Sequence instance. Sequences are now a kind of a grouping attribute for the Shots. And Shots can have more than one Sequence.
Note
New in version 0.2.0.
Shots now have a new attribute called
scenes
, holdingScene
instances which is another grouping attribute likesequences
. Where Sequences are grouping the Shots according to their temporal position to each other, Scenes are grouping the Shots according to their view to the world, that is shots taking place in the same set configuration can be grouped together by using Scenes.Two shots with the same
code
can not be assigned to the sameSequence
.Note
New in version 0.2.10.
Simplified the implementation of
cut_in
,cut_out
andcut_duration
attributes. Thecut_duration
is always the difference betweencut_in
andcut_out
and its value is only be calculated when it is requested. This greatly simplifies the implementation ofcut_in
andcut_out
attributes.The
cut_out
andcut_duration
attributes effects each other. Setting thecut_out
will change thecut_duration
and setting thecut_duration
will change thecut_out
value. The default value of thecut_duration
attribute is calculated from thecut_in
andcut_out
attributes. If bothcut_out
andcut_duration
arguments are set to None, thecut_duration
defaults to 1 andcut_out
will be set tocut_in
+cut_duration
. So the priority of the attributes are as follows:Note
New in version 0.2.4.
handles_at_start
andhandles_at_end
attributes.Note
New in version 0.2.17.2.
Per shot FPS values. It is now possible to change the shot fps by setting its
fps
attribute. The default values is same with theProject
.Parameters: - project (
Project
) – This is theProject
instance that this shot belongs to. A Shot can not be created without a Project instance. - sequences (list of
Sequence
) – This is a list ofSequence
s that this shot is assigned to. A Shot can be created without having a Sequence instance. - cut_in (int) – The in frame number that this shot starts. The default
value is 1. When the
cut_in
is bigger thencut_out
, thecut_out
attribute is set tocut_in
+ 1. - cut_duration (int) – The duration of this shot in frames. It should be zero or a positive integer value (natural number?) or . The default value is None.
- cut_out (int) – The out frame number that this shot ends. If it is
given as a value lower then the
cut_in
parameter, then thecut_out
will be recalculated from the existentcut_in
cut_duration
attributes. Can be skipped. The default value is None. - image_format (
ImageFormat
) – The image format of this shot. This is an optional variable to differentiate the image format per shot. The default value is the same with the Project that this Shot belongs to. - fps (float) – The FPS of this shot. Default value is the same with the
Project
.
-
__init__
(code=None, project=None, sequences=None, scenes=None, cut_in=None, cut_out=None, source_in=None, source_out=None, record_in=None, image_format=None, fps=None, **kwargs)¶
Methods
__init__
([code, project, sequences, scenes, …])create_time_log
(resource, start, end)A helper method to create TimeLogs, this will ease creating TimeLog instances for task. hold
()Pauses the execution of this task by setting its status to OH. least_meaningful_time_unit
(seconds[, …])returns the least meaningful timing unit that corresponds to the request_review
()Creates and returns Review instances for each of the responsible of this task and sets the task status to PREV. request_revision
([reviewer, description, …])Requests revision. resume
()Resumes the execution of this task by setting its status to RTS or WIP depending to its time_logs attribute, so if it has TimeLogs then it will resume as WIP and if it doesn’t then it will resume as RTS. review_set
([review_number])returns the reviews with the given review_number, if review_number round_time
(dt)Round the given datetime object to the defaults.timing_resolution. stop
()Stops this task. to_seconds
(timing, unit, model)converts the schedule values to seconds, depending on to the update_parent_statuses
()updates the parent statuses of this task if any update_schedule_info
()updates the total_logged_seconds and schedule_seconds attributes by update_status_with_children_statuses
()updates the task status according to its children statuses update_status_with_dependent_statuses
([removing])updates the status by looking at the dependent tasks walk_dependencies
([method])Walks the dependencies of this task walk_hierarchy
([method])Walks the hierarchy of this task. Attributes
absolute_path
the absolute_path attribute allocation_strategy
Please read Task
class documentation for details.alternative_resources
The list of User
s assigned to this Task as an alternative resource.bid_timing
The value of the initial bid of this Task. bid_unit
The unit of the initial bid of this Task. children
Other Budget
instances which are the children of this one.code
The code name of this object. computed_duration
returns the computed_duration as the difference of computed_start computed_end
computed_resources
getter for the _computed_resources attribute computed_start
computed_total_seconds
returns the duration as seconds created_by
The User
who has created this object.created_by_id
The id of the User
who has created this entity.cut_duration
getter for the cut_duration property cut_in
The start frame of this shot. cut_out
The end frame of this shot. date_created
A datetime.datetime
instance showing the creation date and time of this object.date_updated
A datetime.datetime
instance showing the update date and time of this object.dependent_of
A descriptor that presents a read/write view of an object attribute. depends
A descriptor that presents a read/write view of an object attribute. description
Description of this object. duration
Duration of the entity. end
overridden end getter entity_groups
entity_id
entity_type
generic_data
This attribute can hold any kind of data which exists in SOM. generic_text
This attribute can hold any text. good
good_id
html_class
html_style
id
image_format
The image_format of this shot. image_format_id
is_container
Returns True if the Task has children Tasks is_leaf
Returns True if the Task has no children Tasks is_milestone
Specifies if this Task is a milestone. is_root
Returns True if the Task has no parent is_scheduled
A predicate which returns True if this task has both a level
Returns the level of this task. metadata
name
Name of this object nice_name
Nice name of this object. notes
All the Notes
s attached to this entity.open_tickets
returns the open tickets referencing this task in their links parent
A Task
instance which is the parent of this Task.parent_id
parents
Returns all of the parents of this mixed in class starting from the path
The path attribute will generate a path suitable for placing the files under it. percent_complete
returns the percent_complete based on the total_logged_seconds and persistent_allocation
Please read Task
class documentation for details.plural_class_name
the plural name of this class priority
An integer number between 0 and 1000 used by TaskJuggler to determine the priority of this Task. project
The owner Project of this task. project_id
The id of the owner Project
of this Task.query
record_in
The start frame in the Editors timeline specifying the start frame general placement of this shot. references
A list of Link
instances given as a reference for this entity.remaining_seconds
returns the remaining amount of efforts, length or duration left resources
The list of User
s assigned to this Task.responsible
The responsible of this task. review_number
returns the _review_number attribute value reviews
A list of Review
holding the details about the reviews created for this task.scenes
schedule_constraint
An integer number showing the constraint schema for this task. schedule_model
Defines the schedule model which is going to be used by TaskJuggler while scheduling this Task. schedule_seconds
returns the total effort, length or duration in seconds, for schedule_timing
It is the value of the schedule timing. schedule_unit
It is the unit of the schedule timing. sequences
shot_id
source_in
The start frame of the used range, should be in between:attr:.cut_in and cut_out
source_out
The end frame of the used range, should be in between:attr:.cut_in and :attr:.cut_out` start
overridden start getter status
The current status of the object. status_id
status_list
status_list_id
tags
A list of tags attached to this object. task_dependent_of
A list of Task
s that this one is being depended by.task_depends_to
A list of Task
s that this one is depending on.task_id
The primary_key
attribute for theTasks
table used by SQLAlchemy to map this Task in relationships.tasks
A synonym for the children
attribute used by the descendants of theTask
class (currentlyAsset
,Shot
andSequence
classes).thumbnail
thumbnail_id
tickets
returns the tickets referencing this task in their links attribute time_logs
A list of TimeLog
instances showing who and when has spent how much effort on this task.tjp_abs_id
returns the calculated absolute id of this task tjp_id
returns TaskJuggler compatible id to_tjp
TaskJuggler representation of this task total_logged_seconds
The total effort spent for this Task. total_seconds
returns the duration as seconds type
The type of the object. type_id
The id of the Type
of this entity.updated_by
The User
who has updated this object.updated_by_id
The id of the User
who has updated this entity.versions
A list of Version
instances showing the files created for this task.watchers
The list of User
s watching this Task.-
cut_in
¶ The start frame of this shot. It is the start frame of the playback range in the application (Maya, Nuke etc.).
-
cut_out
¶ The end frame of this shot. It is the end frame of the playback range in the application (Maya, Nuke etc.).
-
source_out
¶ The end frame of the used range, should be in between:attr:.cut_in and :attr:.cut_out`
-
record_in
¶ The start frame in the Editors timeline specifying the start frame general placement of this shot.
-
absolute_path
¶ the absolute_path attribute
-
bid_timing
¶ The value of the initial bid of this Task. It is an integer or a float.
-
bid_unit
¶ The unit of the initial bid of this Task. It is a string value. And should be one of ‘min’, ‘h’, ‘d’, ‘w’, ‘m’, ‘y’.
-
children
¶ Other
Budget
instances which are the children of this one. This attribute along with theparent
attribute is used in creating a DAG hierarchy of tasks.
-
code
¶ The code name of this object.
It accepts strings. Can not be None.
-
computed_duration
¶ returns the computed_duration as the difference of computed_start and computed_end if there are computed_start and computed_end otherwise returns None
-
computed_resources
¶ getter for the _computed_resources attribute
-
computed_total_seconds
¶ returns the duration as seconds
-
create_time_log
(resource, start, end)¶ A helper method to create TimeLogs, this will ease creating TimeLog instances for task.
-
date_created
¶ A
datetime.datetime
instance showing the creation date and time of this object.
-
date_updated
¶ A
datetime.datetime
instance showing the update date and time of this object.
-
description
¶ Description of this object.
-
duration
¶ Duration of the entity.
It is a datetime.timedelta instance. Showing the difference of the
start
and theend
. If edited it changes theend
attribute value.
-
end
¶ overridden end getter
-
generic_data
¶ This attribute can hold any kind of data which exists in SOM.
-
generic_text
¶ This attribute can hold any text.
-
hold
()¶ Pauses the execution of this task by setting its status to OH. Only applicable to RTS and WIP tasks, any task with other statuses will raise a ValueError.
-
is_container
¶ Returns True if the Task has children Tasks
-
is_leaf
¶ Returns True if the Task has no children Tasks
-
is_milestone
¶ Specifies if this Task is a milestone.
Milestones doesn’t need any duration, any effort and any resources. It is used to create meaningful dependencies between the critical stages of the project.
-
is_root
¶ Returns True if the Task has no parent
-
is_scheduled
¶ A predicate which returns True if this task has both a computed_start and computed_end values
-
least_meaningful_time_unit
(seconds, as_work_time=True)¶ returns the least meaningful timing unit that corresponds to the given seconds. So if:
- as_work_time == True
- seconds % (1 years work time as seconds) == 0 –> ‘y’ else: seconds % (1 month work time as seconds) == 0 –> ‘m’ else: seconds % (1 week work time as seconds) == 0 –> ‘w’ else: seconds % (1 day work time as seconds) == 0 –> ‘d’ else: seconds % (1 hour work time as seconds) == 0 –> ‘h’ else: seconds % (1 minutes work time as seconds) == 0 –> ‘min’ else: raise RuntimeError
- as_work_time == False
- seconds % (1 years as seconds) == 0 –> ‘y’ else: seconds % (1 month as seconds) == 0 –> ‘m’ else: seconds % (1 week as seconds) == 0 –> ‘w’ else: seconds % (1 day as seconds) == 0 –> ‘d’ else: seconds % (1 hour as seconds) == 0 –> ‘h’ else: seconds % (1 minutes as seconds) == 0 –> ‘min’ else: raise RuntimeError
Parameters: Returns int, string: Returns one integer and one string, showing the timing value and the unit.
-
level
¶ Returns the level of this task. It is a temporary property and will be useless when Stalker has its own implementation of a proper Gantt Chart. Write now it is used by the jQueryGantt.
-
name
¶ Name of this object
-
nice_name
¶ Nice name of this object.
It has the same value with the name (contextually) but with a different format like, all the white spaces replaced by underscores (“_”), all the CamelCase form will be expanded by underscore (_) characters and it is always lower case.
-
notes
¶ All the
Notes
s attached to this entity.It is a list of
Note
instances or an empty list, setting it to None will raise a TypeError.
-
open_tickets
¶ returns the open tickets referencing this task in their links attribute
-
parent
¶ A
Task
instance which is the parent of this Task. In Stalker it is possible to create a hierarchy of Task.
-
parents
¶ Returns all of the parents of this mixed in class starting from the root
-
path
¶ The path attribute will generate a path suitable for placing the files under it. It will use the
FilenameTemplate
class related to theProject
Structure
with thetarget_entity_type
is set to the type of this instance.
-
percent_complete
¶ returns the percent_complete based on the total_logged_seconds and schedule_seconds of the task. Container tasks will use info from their children
-
plural_class_name
¶ the plural name of this class
-
priority
¶ An integer number between 0 and 1000 used by TaskJuggler to determine the priority of this Task. The default value is 500.
-
project
¶ The owner Project of this task.
It is a read-only attribute. It is not possible to change the owner Project of a Task it is defined when the Task is created.
-
project_id
¶ The id of the owner
Project
of this Task. This attribute is mainly used by SQLAlchemy to map aProject
instance to a Task.
-
remaining_seconds
¶ returns the remaining amount of efforts, length or duration left in this Task as seconds.
-
request_review
()¶ Creates and returns Review instances for each of the responsible of this task and sets the task status to PREV.
New in version 0.2.0: Request review will not cap the timing of this task anymore.
Only applicable to leaf tasks.
-
request_revision
(reviewer=None, description=”, schedule_timing=1, schedule_unit=’h’)¶ Requests revision.
Applicable to PREV or CMPL leaf tasks. This method will expand the schedule timings of the task according to the supplied arguments.
When request_revision is called on a PREV task, the other NEW Review instances (those created when request_review on a WIP task is called and still waiting a review) will be deleted.
This method at the end will return a new Review instance with correct attributes (reviewer, description, schedule_timing, schedule_unit and review_number attributes).
Parameters: - reviewer (class:.User) – This is the user that requested the revision. He/she doesn’t need to be the responsible, anybody that has a Permission to create a Review instance can request a revision.
- description (str) – The description of the requested revision.
- schedule_timing (int) – The timing value of the requested revision.
The task will be extended this much of duration. Works along with the
schedule_unit
parameter. The default value is 1. - schedule_unit (str) – The timin unit value of the requested
revision. The task will be extended this much of duration. Works
along with the
schedule_timing
parameter. The default value is ‘h’ for ‘hour’.
-
responsible
¶ The responsible of this task.
This attribute will return the responsible of this task which is a list of
User
instances. If there is no responsible set for this task, then it will try to find a responsible in its parents.
-
resume
()¶ Resumes the execution of this task by setting its status to RTS or WIP depending to its time_logs attribute, so if it has TimeLogs then it will resume as WIP and if it doesn’t then it will resume as RTS. Only applicable to Tasks with status OH.
-
review_number
¶ returns the _review_number attribute value
-
review_set
(review_number=None)¶ returns the reviews with the given review_number, if review_number is skipped it will return the latest set of reviews
-
round_time
(dt)¶ Round the given datetime object to the defaults.timing_resolution.
Uses
stalker.defaults.timing_resolution
as the closest number of seconds to round to.Parameters: dt (datetime.datetime) – datetime.datetime object, defaults to now. Based on Thierry Husson’s answer in Stackoverflow
Stackoverflow : http://stackoverflow.com/a/10854034/1431079
-
schedule_constraint
¶ An integer number showing the constraint schema for this task.
Possible values are:
0 Constrain None 1 Constrain Start 2 Constrain End 3 Constrain Both For convenience use stalker.models.task.CONSTRAIN_NONE, stalker.models.task.CONSTRAIN_START, stalker.models.task.CONSTRAIN_END, stalker.models.task.CONSTRAIN_BOTH.
This value is going to be used to constrain the start and end date values of this task. So if you want to pin the start of a task to a certain date. Set its
schedule_constraint
value to CONSTRAIN_START. When the task is scheduled by TaskJuggler the start date will be pinned to thestart
attribute of this task.And if both of the date values (start and end) wanted to be pinned to certain dates (making the task effectively a
duration
task) set the desiredstart
andend
and then set theschedule_constraint
to CONSTRAIN_BOTH.
-
schedule_model
¶ Defines the schedule model which is going to be used by TaskJuggler while scheduling this Task. It has three possible values; effort, duration, length.
effort
is the default value. Each value causes this task to be scheduled in different ways:effort If the schedule_model
attribute is set to “effort” then the start and end date values are calculated so that a resource should spent this much of work time to complete a Task. For example, a task withschedule_timing
of 4 days, needs 4 working days. So it can take 4 working days to complete the Task, but it doesn’t mean that the task duration will be 4 days. If the resource works overtime then the task will be finished before 4 days or if the resource will not be available (due to a vacation) then the task duration can be much more.duration The duration of the task will exactly be equal to schedule_timing
regardless of the resource availability. So the difference betweenstart
andend
attribute values are equal toschedule_timing
. Essentially making the task duration in calendar days instead of working days.length In this model the duration of the task will exactly be equal to the given length value in working days regardless of the resource availability. So a task with the schedule_timing
is set to 4 days will be completed in 4 working days. But again it will not be always 4 calendar days due to the weekends or non working days.
-
schedule_seconds
¶ returns the total effort, length or duration in seconds, for completeness calculation
-
schedule_timing
¶ It is the value of the schedule timing. It is a float value.
The timing value can either be as Work Time or Calendar Time defined by the schedule_model attribute. So when the schedule_model is duration then the value of this attribute is in Calendar Time, and if the schedule_model is either length or effort then the value is considered as Work Time.
-
schedule_unit
¶ It is the unit of the schedule timing. It is a string value. And should be one of ‘min’, ‘h’, ‘d’, ‘w’, ‘m’, ‘y’.
-
start
¶ overridden start getter
-
status
¶ The current status of the object.
It is a
Status
instance which is one of the Statuses stored in thestatus_list
attribute of this object.
-
stop
()¶ Stops this task. It is nearly equivalent to deleting this task. But this will at least preserve the TimeLogs entered for this task. It is only possible to stop WIP tasks.
You can use
resume()
to resume the task.The only difference between
hold()
(other than setting the task to different statuses) is the schedule info, while thehold()
method will preserve the schedule info, stop() will set the schedule info to the current effort.So if 2 days of effort has been entered for a 4 days task, when stopped the task effort will be capped to 2 days, thus TaskJuggler will not try to reserve any resource for this task anymore.
Also, STOP tasks will be ignored in dependency relations.
A list of tags attached to this object.
It is a list of
Tag
instances which shows the tags of this object
-
task_dependent_of
¶ A list of
Task
s that this one is being depended by.A CircularDependencyError will be raised when the task dependency creates a circular dependency which means it is not allowed to create a dependency for this Task which is depending on another one which in some way depends to this one again.
-
task_depends_to
¶ A list of
Task
s that this one is depending on.A CircularDependencyError will be raised when the task dependency creates a circular dependency which means it is not allowed to create a dependency for this Task which is depending on another one which in some way depends to this one again.
-
task_id
¶ The
primary_key
attribute for theTasks
table used by SQLAlchemy to map this Task in relationships.
-
tasks
¶ A synonym for the
children
attribute used by the descendants of theTask
class (currentlyAsset
,Shot
andSequence
classes).
-
tickets
¶ returns the tickets referencing this task in their links attribute
-
tjp_abs_id
¶ returns the calculated absolute id of this task
-
tjp_id
¶ returns TaskJuggler compatible id
-
to_seconds
(timing, unit, model)¶ converts the schedule values to seconds, depending on to the schedule_model the value will differ. So if the schedule_model is ‘effort’ or ‘length’ then the schedule_time and schedule_unit values are interpreted as work time, if the schedule_model is ‘duration’ then the schedule_time and schedule_unit values are considered as calendar time.
-
to_tjp
¶ TaskJuggler representation of this task
-
total_logged_seconds
¶ The total effort spent for this Task. It is the sum of all the TimeLogs recorded for this task as seconds.
Returns int: An integer showing the total seconds spent.
-
total_seconds
¶ returns the duration as seconds
-
type
¶ The type of the object.
It is a
Type
instance with a properType.target_entity_type
.
-
type_id
¶ The id of the
Type
of this entity. Mainly used by SQLAlchemy to create a Many-to-One relates between SimpleEntities and Types.
-
update_parent_statuses
()¶ updates the parent statuses of this task if any
-
update_schedule_info
()¶ updates the total_logged_seconds and schedule_seconds attributes by using the children info and triggers an update on every children
-
update_status_with_children_statuses
()¶ updates the task status according to its children statuses
-
update_status_with_dependent_statuses
(removing=None)¶ updates the status by looking at the dependent tasks
Parameters: removing – The item that is been removing right now, used for the remove event to overcome the update issue.
-
walk_dependencies
(method=1)¶ Walks the dependencies of this task
Parameters: method – The walk method, 0: Depth First, 1: Breadth First
-
walk_hierarchy
(method=0)¶ Walks the hierarchy of this task.
Parameters: method – The walk method, 0: Depth First, 1: Breadth First
-
fps
¶ The fps of this shot.
-
cut_duration
¶ getter for the cut_duration property
-
image_format
¶ The image_format of this shot. Set it to None to re-sync with Project.image_format.
- project (