stalker.models.review.Review¶
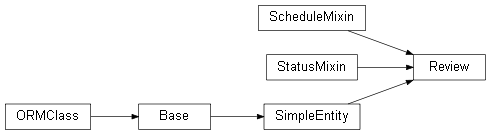
-
class
stalker.models.review.
Review
(task=None, reviewer=None, description=”, **kwargs)[source]¶ Bases:
stalker.models.entity.SimpleEntity
,stalker.models.mixins.ScheduleMixin
,stalker.models.mixins.StatusMixin
Manages the Task Review Workflow.
This class represents a very important part of the review workflow. For more information about the workflow please read the documentation about the Stalker Task Review Workflow.
According to the workflow, Review instances holds information about what have the responsible of the task requested about the task when the resource requested a review from the responsible.
Each Review instance with the same
review_number
for aTask
represents a set of reviews.Creating a review will automatically cap the schedule timing value of the related task to the total logged time logs for that task and then extend the timing values according to the review schedule values.
Parameters: - task (
Task
) – ATask
instance that this review is related to. It can not be skipped. - review_number (int) – This number represents the revision set id that this Review instance belongs to.
- reviewer (
User
) – One of the responsible of the related Task. There will be only one Review instances with the same review_number for every responsible of the same Task. - schedule_timing – Holds the timing value of this review. It is a float value. Only useful if it is a review which ends up requesting a revision.
- schedule_unit – Holds the timing unit of this review. Only useful if it is a review which ends up requesting a revision.
- schedule_model – It holds the schedule model of this review. Only useful if it is a review which ends up requesting a revision.
-
__init__
(task=None, reviewer=None, description=”, **kwargs)¶
Methods
__init__
([task, reviewer, description])approve
()Finalizes the review by approving the task finalize_review_set
()finalizes the current review set Review decisions is_finalized
()A predicate method that checks if all reviews in the same set with least_meaningful_time_unit
(seconds[, …])returns the least meaningful timing unit that corresponds to the request_revision
([schedule_timing, …])Finalizes the review by requesting a revision to_seconds
(timing, unit, model)converts the schedule values to seconds, depending on to the Attributes
created_by
The User
who has created this object.created_by_id
The id of the User
who has created this entity.date_created
A datetime.datetime
instance showing the creation date and time of this object.date_updated
A datetime.datetime
instance showing the update date and time of this object.description
Description of this object. entity_groups
entity_type
generic_data
This attribute can hold any kind of data which exists in SOM. generic_text
This attribute can hold any text. html_class
html_style
id
metadata
name
Name of this object nice_name
Nice name of this object. plural_class_name
the plural name of this class query
review_id
review_number
returns the _review_number attribute value review_set
returns the Review instances in the same review set reviewer
reviewer_id
The User which does the review, also on of the responsible of the related Task schedule_constraint
An integer number showing the constraint schema for this task. schedule_model
Defines the schedule model which is going to be used by TaskJuggler while scheduling this Task. schedule_seconds
Returns the schedule values as seconds, depending on to the schedule_model the value will differ. schedule_timing
It is the value of the schedule timing. schedule_unit
It is the unit of the schedule timing. status
The current status of the object. status_id
status_list
status_list_id
task
The Task
instance that this Review is created fortask_id
The id of the related task. thumbnail
thumbnail_id
tjp_id
returns TaskJuggler compatible id to_tjp
renders a TaskJuggler compliant string used for TaskJuggler type
The type of the object. type_id
The id of the Type
of this entity.updated_by
The User
who has updated this object.updated_by_id
The id of the User
who has updated this entity.-
task_id
¶ The id of the related task.
-
reviewer_id
¶ The User which does the review, also on of the responsible of the related Task
-
status
¶ The current status of the object.
It is a
Status
instance which is one of the Statuses stored in thestatus_list
attribute of this object.
-
review_number
¶ returns the _review_number attribute value
-
review_set
¶ returns the Review instances in the same review set
-
is_finalized
()[source]¶ A predicate method that checks if all reviews in the same set with this one is finalized
-
request_revision
(schedule_timing=1, schedule_unit=’h’, description=”)[source]¶ Finalizes the review by requesting a revision
-
date_created
¶ A
datetime.datetime
instance showing the creation date and time of this object.
-
date_updated
¶ A
datetime.datetime
instance showing the update date and time of this object.
-
description
¶ Description of this object.
-
generic_data
¶ This attribute can hold any kind of data which exists in SOM.
-
generic_text
¶ This attribute can hold any text.
-
least_meaningful_time_unit
(seconds, as_work_time=True)¶ returns the least meaningful timing unit that corresponds to the given seconds. So if:
- as_work_time == True
- seconds % (1 years work time as seconds) == 0 –> ‘y’ else: seconds % (1 month work time as seconds) == 0 –> ‘m’ else: seconds % (1 week work time as seconds) == 0 –> ‘w’ else: seconds % (1 day work time as seconds) == 0 –> ‘d’ else: seconds % (1 hour work time as seconds) == 0 –> ‘h’ else: seconds % (1 minutes work time as seconds) == 0 –> ‘min’ else: raise RuntimeError
- as_work_time == False
- seconds % (1 years as seconds) == 0 –> ‘y’ else: seconds % (1 month as seconds) == 0 –> ‘m’ else: seconds % (1 week as seconds) == 0 –> ‘w’ else: seconds % (1 day as seconds) == 0 –> ‘d’ else: seconds % (1 hour as seconds) == 0 –> ‘h’ else: seconds % (1 minutes as seconds) == 0 –> ‘min’ else: raise RuntimeError
Parameters: Returns int, string: Returns one integer and one string, showing the timing value and the unit.
-
name
¶ Name of this object
-
nice_name
¶ Nice name of this object.
It has the same value with the name (contextually) but with a different format like, all the white spaces replaced by underscores (“_”), all the CamelCase form will be expanded by underscore (_) characters and it is always lower case.
-
plural_class_name
¶ the plural name of this class
-
schedule_constraint
¶ An integer number showing the constraint schema for this task.
Possible values are:
0 Constrain None 1 Constrain Start 2 Constrain End 3 Constrain Both For convenience use stalker.models.task.CONSTRAIN_NONE, stalker.models.task.CONSTRAIN_START, stalker.models.task.CONSTRAIN_END, stalker.models.task.CONSTRAIN_BOTH.
This value is going to be used to constrain the start and end date values of this task. So if you want to pin the start of a task to a certain date. Set its
schedule_constraint
value to CONSTRAIN_START. When the task is scheduled by TaskJuggler the start date will be pinned to thestart
attribute of this task.And if both of the date values (start and end) wanted to be pinned to certain dates (making the task effectively a
duration
task) set the desiredstart
andend
and then set theschedule_constraint
to CONSTRAIN_BOTH.
-
schedule_model
¶ Defines the schedule model which is going to be used by TaskJuggler while scheduling this Task. It has three possible values; effort, duration, length.
effort
is the default value. Each value causes this task to be scheduled in different ways:effort If the schedule_model
attribute is set to “effort” then the start and end date values are calculated so that a resource should spent this much of work time to complete a Task. For example, a task withschedule_timing
of 4 days, needs 4 working days. So it can take 4 working days to complete the Task, but it doesn’t mean that the task duration will be 4 days. If the resource works overtime then the task will be finished before 4 days or if the resource will not be available (due to a vacation) then the task duration can be much more.duration The duration of the task will exactly be equal to schedule_timing
regardless of the resource availability. So the difference betweenstart
andend
attribute values are equal toschedule_timing
. Essentially making the task duration in calendar days instead of working days.length In this model the duration of the task will exactly be equal to the given length value in working days regardless of the resource availability. So a task with the schedule_timing
is set to 4 days will be completed in 4 working days. But again it will not be always 4 calendar days due to the weekends or non working days.
-
schedule_seconds
¶ Returns the schedule values as seconds, depending on to the schedule_model the value will differ. So if the schedule_model is ‘effort’ or ‘length’ then the schedule_time and schedule_unit values are interpreted as work time, if the schedule_model is ‘duration’ then the schedule_time and schedule_unit values are considered as calendar time.
-
schedule_timing
¶ It is the value of the schedule timing. It is a float value.
The timing value can either be as Work Time or Calendar Time defined by the schedule_model attribute. So when the schedule_model is duration then the value of this attribute is in Calendar Time, and if the schedule_model is either length or effort then the value is considered as Work Time.
-
schedule_unit
¶ It is the unit of the schedule timing. It is a string value. And should be one of ‘min’, ‘h’, ‘d’, ‘w’, ‘m’, ‘y’.
-
tjp_id
¶ returns TaskJuggler compatible id
-
to_seconds
(timing, unit, model)¶ converts the schedule values to seconds, depending on to the schedule_model the value will differ. So if the schedule_model is ‘effort’ or ‘length’ then the schedule_time and schedule_unit values are interpreted as work time, if the schedule_model is ‘duration’ then the schedule_time and schedule_unit values are considered as calendar time.
-
to_tjp
¶ renders a TaskJuggler compliant string used for TaskJuggler integration. Needs to be overridden in inherited classes.
-
type
¶ The type of the object.
It is a
Type
instance with a properType.target_entity_type
.
- task (