API Documentation¶
Interpolation¶
interpolation.interpolation |
|
interpolation.no |
|
interpolation.left |
|
interpolation.constant |
|
interpolation.flat |
|
interpolation.right |
|
interpolation.flat |
|
interpolation.nearest |
|
interpolation.zero |
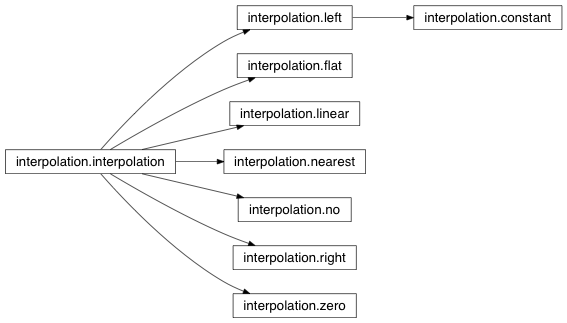
-
class
interpolation.
constant
(x_list=[], y_list=[])[source]¶ Bases:
interpolation.left
-
class
interpolation.
flat
(y=0.0)[source]¶ Bases:
interpolation.interpolation
-
class
interpolation.
left
(x_list=[], y_list=[])[source]¶ Bases:
interpolation.interpolation
-
class
interpolation.
linear
(x_list=[], y_list=[])[source]¶ Bases:
interpolation.interpolation
-
class
interpolation.
nearest
(x_list=[], y_list=[])[source]¶ Bases:
interpolation.interpolation
-
class
interpolation.
no
(x_list=[], y_list=[])[source]¶ Bases:
interpolation.interpolation
-
class
interpolation.
right
(x_list=[], y_list=[])[source]¶ Bases:
interpolation.interpolation
-
class
interpolation.
zero
(x_list=[], y_list=[])[source]¶ Bases:
interpolation.interpolation
Curve¶
curve.Curve |
Curve object to build function |
curve.DateCurve |
|
curve.RateCurve |
|
curve.DiscountFactorCurve |
|
curve.ZeroRateCurve |
|
curve.CashRateCurve |
|
curve.ShortRateCurve |
|
curve.CreditCurve |
generic curve for default probabilities (under construction) |
curve.SurvivalProbabilityCurve |
|
curve.FlatIntensityCurve |
|
curve.ForwardSurvivalRate |
|
curve.HazardRateCurve |
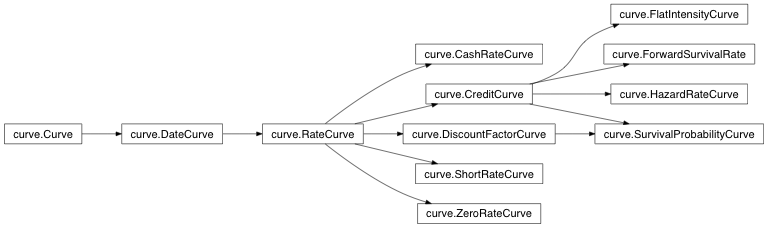
-
class
curve.
CashRateCurve
(x_list, y_list, y_inter=None, origin=None, day_count=None, forward_tenor=None)[source]¶ Bases:
curve.RateCurve
-
class
curve.
CreditCurve
(x_list, y_list, y_inter=None, origin=None, day_count=None, forward_tenor=None)[source]¶ Bases:
curve.RateCurve
generic curve for default probabilities (under construction)
-
class
curve.
Curve
(x_list=None, y_list=None, y_inter=None)[source]¶ Bases:
object
Curve object to build function
Parameters: - x_list (list(float)) – source values
- y_list (list(float)) – target values
- y_inter (list(interpolation.interpolation)) – interpolation function on x_list (optional) or triple of (left, mid, right) interpolation functions with left for x < x_list[0] (as default triple.right is used) right for x > x_list][-1] (as default interpolation.constant is used) mid else (as default interpolation.linear is used)
Curve object to build function f:R→R,x↦y from finite point vectors x and y using piecewise various interpolation functions.
-
domain
¶
-
curve.
DAY_COUNT
(start, end)¶
-
class
curve.
DateCurve
(x_list, y_list, y_inter=None, origin=None, day_count=None)[source]¶ Bases:
curve.Curve
-
domain
¶
-
-
class
curve.
DiscountFactorCurve
(x_list, y_list, y_inter=None, origin=None, day_count=None, forward_tenor=None)[source]¶ Bases:
curve.RateCurve
-
class
curve.
FlatIntensityCurve
(x_list, y_list, y_inter=None, origin=None, day_count=None, forward_tenor=None)[source]¶ Bases:
curve.CreditCurve
-
class
curve.
ForwardSurvivalRate
(x_list, y_list, y_inter=None, origin=None, day_count=None, forward_tenor=None)[source]¶ Bases:
curve.CreditCurve
-
class
curve.
HazardRateCurve
(x_list, y_list, y_inter=None, origin=None, day_count=None, forward_tenor=None)[source]¶ Bases:
curve.CreditCurve
-
class
curve.
RateCurve
(x_list, y_list, y_inter=None, origin=None, day_count=None, forward_tenor=None)[source]¶ Bases:
curve.DateCurve
-
class
curve.
ShortRateCurve
(x_list, y_list, y_inter=None, origin=None, day_count=None, forward_tenor=None)[source]¶ Bases:
curve.RateCurve
Fx Objects¶
fx.FxCurve |
fx rate curve for currency pair |
fx.FxContainer |
FxDict factory object |

-
class
fx.
FxContainer
(currency, domestic_curve)[source]¶ Bases:
dict
FxDict factory object
using triangulation over self.currency defined as a global container of fx information (mainly vs base currency)
today = businessdate() curve = ZeroRateCurve([today], [.02]) container = FxContainer('USD', curve) foreign = ZeroRateCurve([today], [.01]) container.add('EUR', foreign, 1.2) fx_curve = container['USD', 'EUR'] # fx_curve is FxCurve fx_dict = container['USD'] # fx_dict is dict of FxCurves containing fx_curve container['USD']['EUR'](today) == container['USD', 'EUR'](today) # True
Parameters: - currency – base currency of FxContainer
- domestic_curve (RateCurve) – base curve of FxContainer for discounting
-
class
fx.
FxCurve
(x_list, y_list=None, y_inter=None, origin=None, day_count=None, domestic_curve=None, foreign_curve=None)[source]¶ Bases:
curve.DateCurve
fx rate curve for currency pair
Compounding¶
Cashflow¶
cashflow.CashFlowList |
|
cashflow.AmortizingCashFlowList |
|
cashflow.AnnuityCashFlowList |
|
cashflow.RateCashFlowList |
|
cashflow.MultiCashFlowList |
|
cashflow.FixedLoan |
|
cashflow.FloatLoan |
|
cashflow.FixedFloatSwap |
ir swap that pays fixed and receives float. |
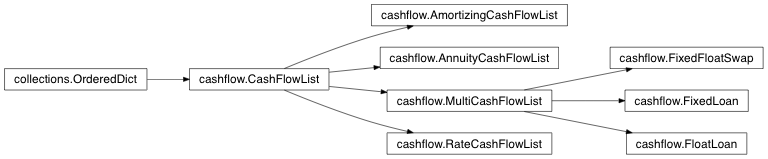
-
class
cashflow.
AmortizingCashFlowList
(pay_date_list, amount_list=None)[source]¶ Bases:
cashflow.CashFlowList
-
class
cashflow.
AnnuityCashFlowList
(pay_date_list, amount_list=None)[source]¶ Bases:
cashflow.CashFlowList
-
class
cashflow.
CashFlowList
(pay_date_list, amount_list=None)[source]¶ Bases:
collections.OrderedDict
-
class
cashflow.
FixedFloatSwap
(date_list, fixed_rate, forward_curve, notional_list=None, day_count=None)[source]¶ Bases:
cashflow.MultiCashFlowList
ir swap that pays fixed and receives float.
-
class
cashflow.
FixedLoan
(legs)[source]¶ Bases:
cashflow.MultiCashFlowList
-
class
cashflow.
FloatLoan
(legs)[source]¶ Bases:
cashflow.MultiCashFlowList
-
class
cashflow.
MultiCashFlowList
(legs)[source]¶ Bases:
cashflow.CashFlowList
-
class
cashflow.
RateCashFlowList
(date_list, day_count, fixed_rate=0.0, forward_curve=None, notional_list=None)[source]¶ Bases:
cashflow.CashFlowList