plotpy.widgets.fit¶
- The fit module provides an interactive curve fitting widget/dialog allowing:
- to fit data manually (by moving sliders)
- or automatically (with standard optimization algorithms
provided by
scipy
).
Example¶
import numpy as np
from plotpy.widgets.fit import FitParam, guifit
def test():
x = np.linspace(-10, 10, 1000)
y = np.cos(1.5*x)+np.random.rand(x.shape[0])*.2
def fit(x, params):
a, b = params
return np.cos(b*x)+a
a = FitParam("Offset", 1., 0., 2.)
b = FitParam("Frequency", 2., 1., 10., logscale=True)
params = [a, b]
values = guifit(x, y, fit, params, xlabel="Time (s)", ylabel="Power (a.u.)")
print(values)
print([param.value for param in params])
if __name__ == "__main__":
test()
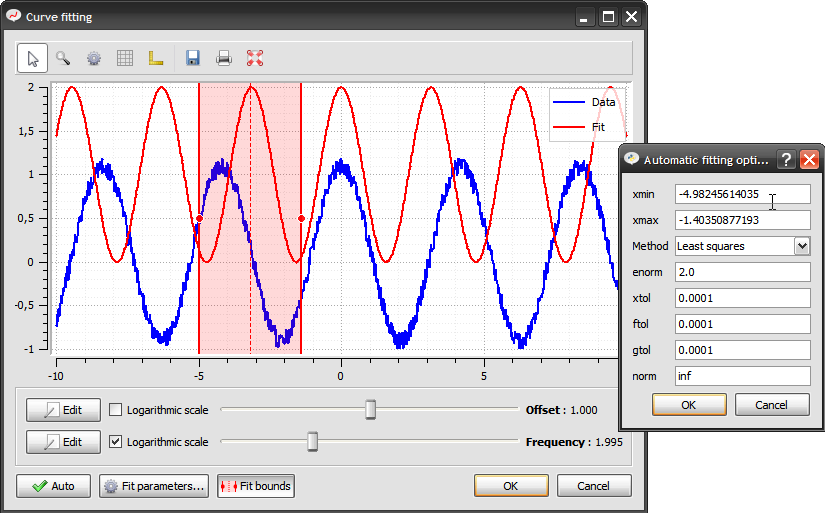
Reference¶
-
plotpy.widgets.fit.
guifit
(x, y, fitfunc, fitparams, fitargs=None, fitkwargs=None, wintitle=None, title=None, xlabel=None, ylabel=None, param_cols=1, auto_fit=True, winsize=None, winpos=None)[source]¶ GUI-based curve fitting tool
-
class
plotpy.widgets.fit.
FitDialog
(wintitle=None, icon='plotpy.svg', edit=True, toolbar=False, options=None, parent=None, panels=None, param_cols=1, legend_anchor='TR', auto_fit=False)[source]¶ -
class
RenderFlags
(QWidget.RenderFlags)¶ QWidget.RenderFlags(int) QWidget.RenderFlags()
-
FitDialog.
accept
()¶
-
FitDialog.
acceptDrops
() → bool¶
-
FitDialog.
accepted
¶ QDialog.accepted [signal]
-
FitDialog.
accessibleDescription
() → str¶
-
FitDialog.
accessibleName
() → str¶
-
FitDialog.
actions
() → list-of-QAction¶
-
FitDialog.
activateWindow
()¶
-
FitDialog.
activate_default_tool
()¶ Activate default tool
-
FitDialog.
addAction
(QAction)¶
-
FitDialog.
addActions
(list-of-QAction)¶
-
FitDialog.
add_panel
(panel)¶ Register a panel to the plot manager
- Plot manager’s registration sequence is the following:
- add plots
- add panels
- add tools
-
FitDialog.
add_plot
(plot, plot_id=<class 'plotpy.plot.DefaultPlotID'>)¶ - Register a plot to the plot manager:
- plot:
plotpy.curve.CurvePlot
orplotpy.image.ImagePlot
object - plot_id (default id is the plot object’s id:
id(plot)
): unique ID identifying the plot (any Python object), this ID will be asked by the manager to access this plot later.
- plot:
- Plot manager’s registration sequence is the following:
- add plots
- add panels
- add tools
-
FitDialog.
add_separator_tool
(toolbar_id=None)¶ Register a separator tool to the plot manager: the separator tool is just a tool which insert a separator in the plot context menu
-
FitDialog.
add_tool
(ToolKlass, *args, **kwargs)¶ - Register a tool to the manager
- ToolKlass: tool’s class (plotpy builtin tools are defined in
module
plotpy.tools
) - args: arguments sent to the tool’s class
- kwargs: keyword arguments sent to the tool’s class
- ToolKlass: tool’s class (plotpy builtin tools are defined in
module
- Plot manager’s registration sequence is the following:
- add plots
- add panels
- add tools
-
FitDialog.
add_toolbar
(toolbar, toolbar_id='default')¶ - Add toolbar to the plot manager
- toolbar: a QToolBar object toolbar_id: toolbar’s id (default id is string “default”)
-
FitDialog.
adjustSize
()¶
-
FitDialog.
autoFillBackground
() → bool¶
-
FitDialog.
backgroundRole
() → QPalette.ColorRole¶
-
FitDialog.
baseSize
() → QSize¶
-
FitDialog.
blockSignals
(bool) → bool¶
-
FitDialog.
childAt
(QPoint) → QWidget¶ QWidget.childAt(int, int) -> QWidget
-
FitDialog.
children
() → list-of-QObject¶
-
FitDialog.
childrenRect
() → QRect¶
-
FitDialog.
childrenRegion
() → QRegion¶
-
FitDialog.
clearFocus
()¶
-
FitDialog.
clearMask
()¶
-
FitDialog.
close
() → bool¶
-
FitDialog.
closeEvent
(QCloseEvent)¶
-
FitDialog.
colorCount
() → int¶
-
FitDialog.
configure_panels
()¶ Call all the registred panels ‘configure_panel’ methods to finalize the object construction (this allows to use tools registered to the same plot manager as the panel itself with breaking the registration sequence: “add plots, then panels, then tools”)
-
FitDialog.
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
FitDialog.
contentsMargins
() → QMargins¶
-
FitDialog.
contentsRect
() → QRect¶
-
FitDialog.
contextMenuEvent
(QContextMenuEvent)¶
-
FitDialog.
contextMenuPolicy
() → Qt.ContextMenuPolicy¶
-
FitDialog.
create_action
(title, triggered=None, toggled=None, shortcut=None, icon=None, tip=None, checkable=None, context=1, enabled=None)¶ Create a new QAction
-
FitDialog.
cursor
() → QCursor¶
-
FitDialog.
customContextMenuRequested
¶ QWidget.customContextMenuRequested[QPoint] [signal]
-
FitDialog.
deleteLater
()¶
-
FitDialog.
depth
() → int¶
-
FitDialog.
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
FitDialog.
devType
() → int¶
-
FitDialog.
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
FitDialog.
done
(int)¶
-
FitDialog.
dumpObjectInfo
()¶
-
FitDialog.
dumpObjectTree
()¶
-
FitDialog.
dynamicPropertyNames
() → list-of-QByteArray¶
-
FitDialog.
effectiveWinId
() → sip.voidptr¶
-
FitDialog.
emit
(SIGNAL(), ...)¶
-
FitDialog.
ensurePolished
()¶
-
FitDialog.
eventFilter
(QObject, QEvent) → bool¶
-
FitDialog.
exec
() → int¶
-
FitDialog.
exec_
() → int¶
-
FitDialog.
extension
() → QWidget¶
-
FitDialog.
find
(sip.voidptr) → QWidget¶
-
FitDialog.
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
FitDialog.
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
FitDialog.
finished
¶ QDialog.finished[int] [signal]
-
FitDialog.
focusPolicy
() → Qt.FocusPolicy¶
-
FitDialog.
focusProxy
() → QWidget¶
-
FitDialog.
focusWidget
() → QWidget¶
-
FitDialog.
font
() → QFont¶
-
FitDialog.
fontInfo
() → QFontInfo¶
-
FitDialog.
fontMetrics
() → QFontMetrics¶
-
FitDialog.
foregroundRole
() → QPalette.ColorRole¶
-
FitDialog.
frameGeometry
() → QRect¶
-
FitDialog.
frameSize
() → QSize¶
-
FitDialog.
geometry
() → QRect¶
-
FitDialog.
getContentsMargins
() -> (int, int, int, int)¶
-
FitDialog.
get_active_plot
()¶ Return the active plot
The active plot is the plot whose canvas has the focus otherwise it’s the “default” plot
-
FitDialog.
get_active_tool
()¶ Return active tool
Return widget context menu – built using active tools
-
FitDialog.
get_contrast_panel
()¶ Convenience function to get the contrast adjustment panel
Return None if the contrast adjustment panel has not been added to this manager
-
FitDialog.
get_default_plot
()¶ Return default plot
The default plot is the plot on which tools and panels will act.
-
FitDialog.
get_default_tool
()¶ Get default tool
-
FitDialog.
get_default_toolbar
()¶ Return default toolbar
-
FitDialog.
get_fitfunc_arguments
()¶ Return fitargs and fitkwargs
-
FitDialog.
get_itemlist_panel
()¶ Convenience function to get the item list panel
Return None if the item list panel has not been added to this manager
-
FitDialog.
get_main
()¶ Return the main (parent) widget
Note that for py:class:plotpy.plot.CurveWidget or
plotpy.plot.ImageWidget
objects, this method will return the widget itself because the plot manager is integrated to it.
-
FitDialog.
get_panel
(panel_id)¶ Return panel from its ID Panel IDs are listed in module plotpy.panels
-
FitDialog.
get_plot
(plot_id=<class 'plotpy.plot.DefaultPlotID'>)¶ Return plot associated to plot_id (if method is called without specifying the plot_id parameter, return the default plot)
-
FitDialog.
get_plots
()¶ Return all registered plots
-
FitDialog.
get_tool
(ToolKlass)¶ Return tool instance from its class
-
FitDialog.
get_toolbar
(toolbar_id='default')¶ - Return toolbar from its ID
- toolbar_id: toolbar’s id (default id is string “default”)
-
FitDialog.
get_values
()¶ Convenience method to get fit parameter values
-
FitDialog.
get_xcs_panel
()¶ Convenience function to get the X-axis cross section panel
Return None if the X-axis cross section panel has not been added to this manager
-
FitDialog.
get_ycs_panel
()¶ Convenience function to get the Y-axis cross section panel
Return None if the Y-axis cross section panel has not been added to this manager
-
FitDialog.
grabGesture
(Qt.GestureType, Qt.GestureFlags flags=Qt.GestureFlags(0))¶
-
FitDialog.
grabKeyboard
()¶
-
FitDialog.
grabMouse
()¶ QWidget.grabMouse(QCursor)
-
FitDialog.
grabShortcut
(QKeySequence, Qt.ShortcutContext context=Qt.WindowShortcut) → int¶
-
FitDialog.
graphicsEffect
() → QGraphicsEffect¶
-
FitDialog.
graphicsProxyWidget
() → QGraphicsProxyWidget¶
-
FitDialog.
hasFocus
() → bool¶
-
FitDialog.
hasMouseTracking
() → bool¶
-
FitDialog.
height
() → int¶
-
FitDialog.
heightForWidth
(int) → int¶
-
FitDialog.
heightMM
() → int¶
-
FitDialog.
hide
()¶
-
FitDialog.
inherits
(str) → bool¶
-
FitDialog.
inputContext
() → QInputContext¶
-
FitDialog.
inputMethodHints
() → Qt.InputMethodHints¶
-
FitDialog.
inputMethodQuery
(Qt.InputMethodQuery) → object¶
-
FitDialog.
insertAction
(QAction, QAction)¶
-
FitDialog.
insertActions
(QAction, list-of-QAction)¶
-
FitDialog.
installEventFilter
(QObject)¶
-
FitDialog.
isActiveWindow
() → bool¶
-
FitDialog.
isAncestorOf
(QWidget) → bool¶
-
FitDialog.
isEnabled
() → bool¶
-
FitDialog.
isEnabledTo
(QWidget) → bool¶
-
FitDialog.
isEnabledToTLW
() → bool¶
-
FitDialog.
isFullScreen
() → bool¶
-
FitDialog.
isHidden
() → bool¶
-
FitDialog.
isLeftToRight
() → bool¶
-
FitDialog.
isMaximized
() → bool¶
-
FitDialog.
isMinimized
() → bool¶
-
FitDialog.
isModal
() → bool¶
-
FitDialog.
isRightToLeft
() → bool¶
-
FitDialog.
isSizeGripEnabled
() → bool¶
-
FitDialog.
isTopLevel
() → bool¶
-
FitDialog.
isVisible
() → bool¶
-
FitDialog.
isVisibleTo
(QWidget) → bool¶
-
FitDialog.
isWidgetType
() → bool¶
-
FitDialog.
isWindow
() → bool¶
-
FitDialog.
isWindowModified
() → bool¶
-
FitDialog.
keyPressEvent
(QKeyEvent)¶
-
FitDialog.
keyboardGrabber
() → QWidget¶
-
FitDialog.
killTimer
(int)¶
-
FitDialog.
layout
() → QLayout¶
-
FitDialog.
layoutDirection
() → Qt.LayoutDirection¶
-
FitDialog.
locale
() → QLocale¶
-
FitDialog.
logicalDpiX
() → int¶
-
FitDialog.
logicalDpiY
() → int¶
-
FitDialog.
lower
()¶
-
FitDialog.
mapFrom
(QWidget, QPoint) → QPoint¶
-
FitDialog.
mapFromGlobal
(QPoint) → QPoint¶
-
FitDialog.
mapFromParent
(QPoint) → QPoint¶
-
FitDialog.
mapTo
(QWidget, QPoint) → QPoint¶
-
FitDialog.
mapToGlobal
(QPoint) → QPoint¶
-
FitDialog.
mapToParent
(QPoint) → QPoint¶
-
FitDialog.
mask
() → QRegion¶
-
FitDialog.
maximumHeight
() → int¶
-
FitDialog.
maximumSize
() → QSize¶
-
FitDialog.
maximumWidth
() → int¶
-
FitDialog.
metaObject
() → QMetaObject¶
-
FitDialog.
minimumHeight
() → int¶
-
FitDialog.
minimumSize
() → QSize¶
-
FitDialog.
minimumSizeHint
() → QSize¶
-
FitDialog.
minimumWidth
() → int¶
-
FitDialog.
mouseGrabber
() → QWidget¶
-
FitDialog.
move
(QPoint)¶ QWidget.move(int, int)
-
FitDialog.
moveToThread
(QThread)¶
-
FitDialog.
nativeParentWidget
() → QWidget¶
-
FitDialog.
nextInFocusChain
() → QWidget¶
-
FitDialog.
normalGeometry
() → QRect¶
-
FitDialog.
numColors
() → int¶
-
FitDialog.
objectName
() → str¶
-
FitDialog.
open
()¶
-
FitDialog.
orientation
() → Qt.Orientation¶
-
FitDialog.
overrideWindowFlags
(Qt.WindowFlags)¶
-
FitDialog.
overrideWindowState
(Qt.WindowStates)¶
-
FitDialog.
paintEngine
() → QPaintEngine¶
-
FitDialog.
paintingActive
() → bool¶
-
FitDialog.
palette
() → QPalette¶
-
FitDialog.
parent
() → QObject¶
-
FitDialog.
parentWidget
() → QWidget¶
-
FitDialog.
physicalDpiX
() → int¶
-
FitDialog.
physicalDpiY
() → int¶
-
FitDialog.
pos
() → QPoint¶
-
FitDialog.
previousInFocusChain
() → QWidget¶
-
FitDialog.
property
(str) → object¶
-
FitDialog.
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
FitDialog.
raise_
()¶
-
FitDialog.
rect
() → QRect¶
-
FitDialog.
refresh
(slider_value=None)¶ Refresh Fit Tool dialog box
-
FitDialog.
register_all_curve_tools
()¶ Register standard, curve-related and other tools
-
FitDialog.
register_all_image_tools
()¶ Register standard, image-related and other tools
-
FitDialog.
register_curve_tools
()¶ Register only curve-related tools
-
FitDialog.
register_image_tools
()¶ Register only image-related tools
-
FitDialog.
register_other_tools
()¶ Register other common tools
-
FitDialog.
register_standard_tools
()¶ Registering basic tools for standard plot dialog –> top of the context-menu
-
FitDialog.
register_tools
()¶ Register the plotting dialog box tools: the base implementation provides standard, curve-related and other tools - i.e. calling this method is exactly the same as calling
plotpy.plot.CurveDialog.register_all_curve_tools()
This method may be overriden to provide a fully customized set of tools
-
FitDialog.
reject
()¶
-
FitDialog.
rejected
¶ QDialog.rejected [signal]
-
FitDialog.
releaseKeyboard
()¶
-
FitDialog.
releaseMouse
()¶
-
FitDialog.
releaseShortcut
(int)¶
-
FitDialog.
removeAction
(QAction)¶
-
FitDialog.
removeEventFilter
(QObject)¶
-
FitDialog.
render
(QPaintDevice, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren)¶ QWidget.render(QPainter, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren)
-
FitDialog.
repaint
()¶ QWidget.repaint(int, int, int, int) QWidget.repaint(QRect) QWidget.repaint(QRegion)
-
FitDialog.
resize
(QSize)¶ QWidget.resize(int, int)
-
FitDialog.
resizeEvent
(QResizeEvent)¶
-
FitDialog.
restoreGeometry
(QByteArray) → bool¶
-
FitDialog.
result
() → int¶
-
FitDialog.
saveGeometry
() → QByteArray¶
-
FitDialog.
scroll
(int, int)¶ QWidget.scroll(int, int, QRect)
-
FitDialog.
setAcceptDrops
(bool)¶
-
FitDialog.
setAccessibleDescription
(str)¶
-
FitDialog.
setAccessibleName
(str)¶
-
FitDialog.
setAttribute
(Qt.WidgetAttribute, bool on=True)¶
-
FitDialog.
setAutoFillBackground
(bool)¶
-
FitDialog.
setBackgroundRole
(QPalette.ColorRole)¶
-
FitDialog.
setBaseSize
(int, int)¶ QWidget.setBaseSize(QSize)
-
FitDialog.
setContentsMargins
(int, int, int, int)¶ QWidget.setContentsMargins(QMargins)
-
FitDialog.
setContextMenuPolicy
(Qt.ContextMenuPolicy)¶
-
FitDialog.
setCursor
(QCursor)¶
-
FitDialog.
setDisabled
(bool)¶
-
FitDialog.
setEnabled
(bool)¶
-
FitDialog.
setExtension
(QWidget)¶
-
FitDialog.
setFixedHeight
(int)¶
-
FitDialog.
setFixedSize
(QSize)¶ QWidget.setFixedSize(int, int)
-
FitDialog.
setFixedWidth
(int)¶
-
FitDialog.
setFocus
()¶ QWidget.setFocus(Qt.FocusReason)
-
FitDialog.
setFocusPolicy
(Qt.FocusPolicy)¶
-
FitDialog.
setFocusProxy
(QWidget)¶
-
FitDialog.
setFont
(QFont)¶
-
FitDialog.
setForegroundRole
(QPalette.ColorRole)¶
-
FitDialog.
setGeometry
(QRect)¶ QWidget.setGeometry(int, int, int, int)
-
FitDialog.
setGraphicsEffect
(QGraphicsEffect)¶
-
FitDialog.
setHidden
(bool)¶
-
FitDialog.
setInputContext
(QInputContext)¶
-
FitDialog.
setInputMethodHints
(Qt.InputMethodHints)¶
-
FitDialog.
setLayout
(QLayout)¶
-
FitDialog.
setLayoutDirection
(Qt.LayoutDirection)¶
-
FitDialog.
setLocale
(QLocale)¶
-
FitDialog.
setMask
(QBitmap)¶ QWidget.setMask(QRegion)
-
FitDialog.
setMaximumHeight
(int)¶
-
FitDialog.
setMaximumSize
(int, int)¶ QWidget.setMaximumSize(QSize)
-
FitDialog.
setMaximumWidth
(int)¶
-
FitDialog.
setMinimumHeight
(int)¶
-
FitDialog.
setMinimumSize
(int, int)¶ QWidget.setMinimumSize(QSize)
-
FitDialog.
setMinimumWidth
(int)¶
-
FitDialog.
setModal
(bool)¶
-
FitDialog.
setMouseTracking
(bool)¶
-
FitDialog.
setObjectName
(str)¶
-
FitDialog.
setOrientation
(Qt.Orientation)¶
-
FitDialog.
setPalette
(QPalette)¶
-
FitDialog.
setParent
(QWidget)¶ QWidget.setParent(QWidget, Qt.WindowFlags)
-
FitDialog.
setProperty
(str, object) → bool¶
-
FitDialog.
setResult
(int)¶
-
FitDialog.
setShortcutAutoRepeat
(int, bool enabled=True)¶
-
FitDialog.
setShortcutEnabled
(int, bool enabled=True)¶
-
FitDialog.
setShown
(bool)¶
-
FitDialog.
setSizeGripEnabled
(bool)¶
-
FitDialog.
setSizeIncrement
(int, int)¶ QWidget.setSizeIncrement(QSize)
-
FitDialog.
setSizePolicy
(QSizePolicy)¶ QWidget.setSizePolicy(QSizePolicy.Policy, QSizePolicy.Policy)
-
FitDialog.
setStatusTip
(str)¶
-
FitDialog.
setStyle
(QStyle)¶
-
FitDialog.
setStyleSheet
(str)¶
-
FitDialog.
setTabOrder
(QWidget, QWidget)¶
-
FitDialog.
setToolTip
(str)¶
-
FitDialog.
setUpdatesEnabled
(bool)¶
-
FitDialog.
setVisible
(bool)¶
-
FitDialog.
setWhatsThis
(str)¶
-
FitDialog.
setWindowFilePath
(str)¶
-
FitDialog.
setWindowFlags
(Qt.WindowFlags)¶
-
FitDialog.
setWindowIcon
(QIcon)¶
-
FitDialog.
setWindowIconText
(str)¶
-
FitDialog.
setWindowModality
(Qt.WindowModality)¶
-
FitDialog.
setWindowModified
(bool)¶
-
FitDialog.
setWindowOpacity
(float)¶
-
FitDialog.
setWindowRole
(str)¶
-
FitDialog.
setWindowState
(Qt.WindowStates)¶
-
FitDialog.
setWindowTitle
(str)¶
-
FitDialog.
set_active_tool
(tool=None)¶ Set active tool (if tool argument is None, the active tool will be the default tool)
-
FitDialog.
set_contrast_range
(zmin, zmax)¶ Convenience function to set the contrast adjustment panel range
This is strictly equivalent to the following:
# Here, *widget* is for example a CurveWidget instance # (the same apply for CurvePlot, ImageWidget, ImagePlot or any # class deriving from PlotManager) widget.get_contrast_panel().set_range(zmin, zmax)
-
FitDialog.
set_default_plot
(plot)¶ Set default plot
The default plot is the plot on which tools and panels will act.
-
FitDialog.
set_default_tool
(tool)¶ Set default tool
-
FitDialog.
set_default_toolbar
(toolbar)¶ Set default toolbar
-
FitDialog.
show
()¶
-
FitDialog.
showEvent
(QShowEvent)¶
-
FitDialog.
showExtension
(bool)¶
-
FitDialog.
showFullScreen
()¶
-
FitDialog.
showMaximized
()¶
-
FitDialog.
showMinimized
()¶
-
FitDialog.
showNormal
()¶
-
FitDialog.
signalsBlocked
() → bool¶
-
FitDialog.
size
() → QSize¶
-
FitDialog.
sizeHint
() → QSize¶
-
FitDialog.
sizeIncrement
() → QSize¶
-
FitDialog.
sizePolicy
() → QSizePolicy¶
-
FitDialog.
stackUnder
(QWidget)¶
-
FitDialog.
startTimer
(int) → int¶
-
FitDialog.
statusTip
() → str¶
-
FitDialog.
style
() → QStyle¶
-
FitDialog.
styleSheet
() → str¶
-
FitDialog.
testAttribute
(Qt.WidgetAttribute) → bool¶
-
FitDialog.
thread
() → QThread¶
-
FitDialog.
toolTip
() → str¶
-
FitDialog.
topLevelWidget
() → QWidget¶
-
FitDialog.
tr
(str, str disambiguation=None, int n=-1) → str¶
-
FitDialog.
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
FitDialog.
underMouse
() → bool¶
-
FitDialog.
ungrabGesture
(Qt.GestureType)¶
-
FitDialog.
unsetCursor
()¶
-
FitDialog.
unsetLayoutDirection
()¶
-
FitDialog.
unsetLocale
()¶
-
FitDialog.
update
()¶ QWidget.update(QRect) QWidget.update(QRegion) QWidget.update(int, int, int, int)
-
FitDialog.
updateGeometry
()¶
-
FitDialog.
update_cross_sections
()¶ Convenience function to update the cross section panels at once
This is strictly equivalent to the following:
# Here, *widget* is for example a CurveWidget instance # (the same apply for CurvePlot, ImageWidget, ImagePlot or any # class deriving from PlotManager) widget.get_xcs_panel().update_plot() widget.get_ycs_panel().update_plot()
-
FitDialog.
update_tools_status
(plot=None)¶ Update tools for current plot
-
FitDialog.
updatesEnabled
() → bool¶
-
FitDialog.
visibleRegion
() → QRegion¶
-
FitDialog.
whatsThis
() → str¶
-
FitDialog.
width
() → int¶
-
FitDialog.
widthMM
() → int¶
-
FitDialog.
winId
() → sip.voidptr¶
-
FitDialog.
window
() → QWidget¶
-
FitDialog.
windowFilePath
() → str¶
-
FitDialog.
windowFlags
() → Qt.WindowFlags¶
-
FitDialog.
windowIcon
() → QIcon¶
-
FitDialog.
windowIconText
() → str¶
-
FitDialog.
windowModality
() → Qt.WindowModality¶
-
FitDialog.
windowOpacity
() → float¶
-
FitDialog.
windowRole
() → str¶
-
FitDialog.
windowState
() → Qt.WindowStates¶
-
FitDialog.
windowTitle
() → str¶
-
FitDialog.
windowType
() → Qt.WindowType¶
-
FitDialog.
x
() → int¶
-
FitDialog.
y
() → int¶
-
class
-
class
plotpy.widgets.fit.
FitParam
(name, value, min, max, logscale=False, steps=5000, format='%.3f', size_offset=0, unit='')[source]¶
-
class
plotpy.widgets.fit.
AutoFitParam
(title=None, comment=None, icon='')[source]¶ -
accept
(vis)¶ helper function that passes the visitor to the accept methods of all the items in this dataset
-
check
()¶ Check the dataset item values
-
edit
(parent=None, apply=None, size=None)¶ - Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
-
get_comment
()¶ Return data set comment
-
get_icon
()¶ Return data set icon
-
get_title
()¶ Return data set title
-
set_defaults
()¶ Set default values
-
text_edit
()¶ Edit data set with text input only
-
to_string
(debug=False, indent=None, align=False)¶ Return readable string representation of the data set If debug is True, add more details on data items
-
view
(parent=None, size=None)¶ - Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
-