stalker.models.studio.Studio¶
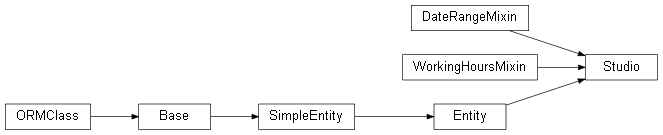
-
class
stalker.models.studio.
Studio
(daily_working_hours=None, now=None, timing_resolution=None, **kwargs)[source]¶ Bases:
stalker.models.entity.Entity
,stalker.models.mixins.DateRangeMixin
,stalker.models.mixins.WorkingHoursMixin
Manage all the studio information at once.
With Stalker you can manage all you Studio data by using this class. Studio knows all the projects, all the departments, all the users and every thing about the studio. But the most important part of the Studio is that it can schedule all the Projects by using TaskJuggler.
Studio class is kind of the database itself:
studio = Studio() # simple data studio.projects studio.active_projects studio.inactive_projects studio.departments studio.users # project management studio.to_tjp # a tjp representation of the studio with all # its projects, departments and resources etc. studio.schedule() # schedules all the active projects at once
Working Hours
In Stalker, Studio class also manages the working hours of the studio. Allowing project tasks to be scheduled to be scheduled in those hours.
Vacations
Studio wide vacations are managed by the Studio class.
Scheduling
There are a couple of attributes those become pretty interesting when used together with the Studio instance while using the scheduling part of the Studio. Please refer to the attribute documentation for each attribute:
Parameters: - daily_working_hours (int) – An integer specifying the daily working hours for the studio. It is another critical value attribute which TaskJuggler uses mainly converting working day values to working hours (1d = 10h kind of thing).
- now (datetime.datetime) – The now attribute overrides the TaskJugglers
now
attribute allowing the user to schedule the projects as if the scheduling is done on that date. The default value is the rounded value of datetime.datetime.now(pytz.utc). - timing_resolution (datetime.timedelta) – The timing_resolution of the datetime.datetime
object in datetime.timedelta. Uses
timing_resolution
settings in thestalker.config.Config
class which defaults to 1 hour. Setting the timing_resolution to less then 5 minutes is not suggested because it is a limit for TaskJuggler.
-
__init__
(daily_working_hours=None, now=None, timing_resolution=None, **kwargs)¶
Methods
__init__
([daily_working_hours, now, …])round_time
(dt)Round the given datetime object to the defaults.timing_resolution. schedule
([scheduled_by])Schedules all the active projects in the studio. to_unit
(from_timing, from_unit, to_unit[, …])converts the given timing and unit to the desired unit update_defaults
()updates the default values with the studio Attributes
active_projects
returns all the active projects in the studio computed_duration
returns the computed_duration as the difference of computed_start computed_end
computed_start
computed_total_seconds
returns the duration as seconds created_by
The User
who has created this object.created_by_id
The id of the User
who has created this entity.daily_working_hours
a shortcut for Studio.working_hours.daily_working_hours date_created
A datetime.datetime
instance showing the creation date and time of this object.date_updated
A datetime.datetime
instance showing the update date and time of this object.departments
returns all the departments in the studio description
Description of this object. duration
Duration of the entity. end
The date that the entity should be delivered. entity_groups
entity_id
entity_type
generic_data
This attribute can hold any kind of data which exists in SOM. generic_text
This attribute can hold any text. html_class
html_style
id
inactive_projects
return all the inactive projects in the studio is_scheduling
is_scheduling_by
The User who is scheduling the Studio projects right now is_scheduling_by_id
The id of the user who is scheduling the Studio projects right now last_schedule_message
Holds the last schedule message, generally coming generated by TaskJuggler last_scheduled_at
Stores the last schedule date last_scheduled_by
The User who has last scheduled the Studio projects last_scheduled_by_id
The id of the user who has last scheduled the Studio projects metadata
name
Name of this object nice_name
Nice name of this object. notes
All the Notes
s attached to this entity.now
now getter plural_class_name
the plural name of this class projects
returns all the projects in the studio query
scheduler
scheduler getter scheduling_started_at
Stores when the current scheduling is started at, it is a good measure for measuring if the last schedule is not correctly finished start
The date that this entity should start. studio_id
tags
A list of tags attached to this object. thumbnail
thumbnail_id
timing_resolution
The timing_resolution of this object. tjp_id
returns TaskJuggler compatible id to_tjp
converts the studio to a tjp representation total_seconds
returns the duration as seconds type
The type of the object. type_id
The id of the Type
of this entity.updated_by
The User
who has updated this object.updated_by_id
The id of the User
who has updated this entity.users
returns all the users in the studio vacations
returns all Vacations which doesn’t have a User defined weekly_working_days
returns the WorkingHours.weekly_working_hours weekly_working_hours
returns the WorkingHours.weekly_working_hours working_hours
yearly_working_days
returns the yearly working days -
is_scheduling_by_id
¶ The id of the user who is scheduling the Studio projects right now
-
is_scheduling_by
¶ The User who is scheduling the Studio projects right now
-
scheduling_started_at
¶ Stores when the current scheduling is started at, it is a good measure for measuring if the last schedule is not correctly finished
-
last_scheduled_at
¶ Stores the last schedule date
-
last_scheduled_by_id
¶ The id of the user who has last scheduled the Studio projects
-
last_scheduled_by
¶ The User who has last scheduled the Studio projects
-
last_schedule_message
¶ Holds the last schedule message, generally coming generated by TaskJuggler
-
daily_working_hours
¶ a shortcut for Studio.working_hours.daily_working_hours
-
now
¶ now getter
-
scheduler
¶ scheduler getter
-
to_tjp
¶ converts the studio to a tjp representation
-
projects
¶ returns all the projects in the studio
-
active_projects
¶ returns all the active projects in the studio
-
inactive_projects
¶ return all the inactive projects in the studio
-
departments
¶ returns all the departments in the studio
-
users
¶ returns all the users in the studio
-
vacations
¶ returns all Vacations which doesn’t have a User defined
-
schedule
(scheduled_by=None)[source]¶ Schedules all the active projects in the studio. Needs a Scheduler, so before calling it set a scheduler by using the
scheduler
attribute.Parameters: scheduled_by – A User instance who is doing the scheduling.
-
weekly_working_hours
¶ returns the WorkingHours.weekly_working_hours
-
weekly_working_days
¶ returns the WorkingHours.weekly_working_hours
-
yearly_working_days
¶ returns the yearly working days
-
to_unit
(from_timing, from_unit, to_unit, working_hours=True)[source]¶ converts the given timing and unit to the desired unit if working_hours=True then the given timing is considered as working hours
-
timing_resolution
¶ The timing_resolution of this object.
Can be set to any value that is representable with datetime.timedelta. The default value is 1 hour. Whenever it is changed the start, end and duration values will be updated.
-
computed_duration
¶ returns the computed_duration as the difference of computed_start and computed_end if there are computed_start and computed_end otherwise returns None
-
computed_total_seconds
¶ returns the duration as seconds
-
date_created
¶ A
datetime.datetime
instance showing the creation date and time of this object.
-
date_updated
¶ A
datetime.datetime
instance showing the update date and time of this object.
-
description
¶ Description of this object.
-
duration
¶ Duration of the entity.
It is a datetime.timedelta instance. Showing the difference of the
start
and theend
. If edited it changes theend
attribute value.
-
end
¶ The date that the entity should be delivered.
The end can be set to a datetime.timedelta and in this case it will be calculated as an offset from the start and converted to datetime.datetime again. Setting the start to a date passing the end will also set the end, so the timedelta between them is preserved, default value is 10 days
-
generic_data
¶ This attribute can hold any kind of data which exists in SOM.
-
generic_text
¶ This attribute can hold any text.
-
name
¶ Name of this object
-
nice_name
¶ Nice name of this object.
It has the same value with the name (contextually) but with a different format like, all the white spaces replaced by underscores (“_”), all the CamelCase form will be expanded by underscore (_) characters and it is always lower case.
-
notes
¶ All the
Notes
s attached to this entity.It is a list of
Note
instances or an empty list, setting it to None will raise a TypeError.
-
plural_class_name
¶ the plural name of this class
-
round_time
(dt)¶ Round the given datetime object to the defaults.timing_resolution.
Uses
stalker.defaults.timing_resolution
as the closest number of seconds to round to.Parameters: dt (datetime.datetime) – datetime.datetime object, defaults to now. Based on Thierry Husson’s answer in Stackoverflow
Stackoverflow : http://stackoverflow.com/a/10854034/1431079
-
start
¶ The date that this entity should start.
Also effects the
DateRangeMixin.end
attribute value in certain conditions, if theDateRangeMixin.start
is set to a time passing theDateRangeMixin.end
it will also offset theDateRangeMixin.end
to keep theDateRangeMixin.duration
value fixed.DateRangeMixin.start
should be an instance of class:datetime.datetime and the default value isdatetime.datetime.now(pytz.utc)()
A list of tags attached to this object.
It is a list of
Tag
instances which shows the tags of this object
-
tjp_id
¶ returns TaskJuggler compatible id
-
total_seconds
¶ returns the duration as seconds
-
type
¶ The type of the object.
It is a
Type
instance with a properType.target_entity_type
.
-
type_id
¶ The id of the
Type
of this entity. Mainly used by SQLAlchemy to create a Many-to-One relates between SimpleEntities and Types.
-
working_hours_id
¶ the id of the related working hours