plotpy.tools¶
- The tools module provides a collection of plot tools :
plotpy.tools.RectZoomTool
plotpy.tools.SelectTool
plotpy.tools.SelectPointTool
plotpy.tools.MultiLineTool
plotpy.tools.FreeFormTool
plotpy.tools.LabelTool
plotpy.tools.RectangleTool
plotpy.tools.PointTool
plotpy.tools.SegmentTool
plotpy.tools.CircleTool
plotpy.tools.EllipseTool
plotpy.tools.PlaceAxesTool
plotpy.tools.AnnotatedRectangleTool
plotpy.tools.AnnotatedCircleTool
plotpy.tools.AnnotatedEllipseTool
plotpy.tools.AnnotatedPointTool
plotpy.tools.AnnotatedSegmentTool
plotpy.tools.HRangeTool
plotpy.tools.DummySeparatorTool
plotpy.tools.AntiAliasingTool
plotpy.tools.DisplayCoordsTool
plotpy.tools.ReverseYAxisTool
plotpy.tools.AspectRatioTool
plotpy.tools.PanelTool
plotpy.tools.ItemListPanelTool
plotpy.tools.ContrastPanelTool
plotpy.tools.ColormapTool
plotpy.tools.XCSPanelTool
plotpy.tools.YCSPanelTool
plotpy.tools.CrossSectionTool
plotpy.tools.AverageCrossSectionTool
plotpy.tools.SaveAsTool
plotpy.tools.CopyToClipboardTool
plotpy.tools.OpenFileTool
plotpy.tools.OpenImageTool
plotpy.tools.SnapshotTool
plotpy.tools.PrintTool
plotpy.tools.SaveItemsTool
plotpy.tools.LoadItemsTool
plotpy.tools.AxisScaleTool
plotpy.tools.HelpTool
plotpy.tools.ExportItemDataTool
plotpy.tools.EditItemDataTool
plotpy.tools.ItemCenterTool
plotpy.tools.DeleteItemTool
A plot tool is an object providing various features to a plotting widget
(plotpy.curve.CurvePlot
or plotpy.image.ImagePlot
):
buttons, menus, selection tools, image I/O tools, etc.
To make it work, a tool has to be registered to the plotting widget’s manager,
i.e. an instance of the plotpy.plot.PlotManager
class (see
the plotpy.plot
module for more details on the procedure).
The CurvePlot and ImagePlot widgets do not provide any PlotManager: the
manager has to be created separately. On the contrary, the ready-to-use widgets
plotpy.plot.CurveWidget
and plotpy.plot.ImageWidget
are higher-level plotting widgets with integrated manager, tools and panels.
See also
- Module
plotpy.plot
- Module providing ready-to-use curve and image plotting widgets and dialog boxes
- Module
plotpy.curve
- Module providing curve-related plot items and plotting widgets
- Module
plotpy.image
- Module providing image-related plot items and plotting widgets
Example¶
The following example add all the existing tools to an ImageWidget object for testing purpose:
import os.path as osp
from plotpy.plot import ImageDialog
from plotpy.tools import (RectangleTool, EllipseTool, HRangeTool, PlaceAxesTool,
MultiLineTool, FreeFormTool, SegmentTool, CircleTool,
AnnotatedRectangleTool, AnnotatedEllipseTool,
AnnotatedSegmentTool, AnnotatedCircleTool, LabelTool,
AnnotatedPointTool,
VCursorTool, HCursorTool, XCursorTool,
ObliqueRectangleTool, AnnotatedObliqueRectangleTool)
from plotpy.builder import make
def create_window():
win = ImageDialog(edit=False, toolbar=True,
wintitle="All image and plot tools test")
for toolklass in (LabelTool, HRangeTool,
VCursorTool, HCursorTool, XCursorTool,
SegmentTool, RectangleTool, ObliqueRectangleTool,
CircleTool, EllipseTool,
MultiLineTool, FreeFormTool, PlaceAxesTool,
AnnotatedRectangleTool, AnnotatedObliqueRectangleTool,
AnnotatedCircleTool, AnnotatedEllipseTool,
AnnotatedSegmentTool, AnnotatedPointTool):
win.add_tool(toolklass)
return win
def test():
"""Test"""
# -- Create QApplication
import guidata
_app = guidata.qapplication()
# --
filename = osp.join(osp.dirname(__file__), "brain.png")
win = create_window()
image = make.image(filename=filename, colormap="bone")
plot = win.get_plot()
plot.add_item(image)
win.exec_()
if __name__ == "__main__":
test()
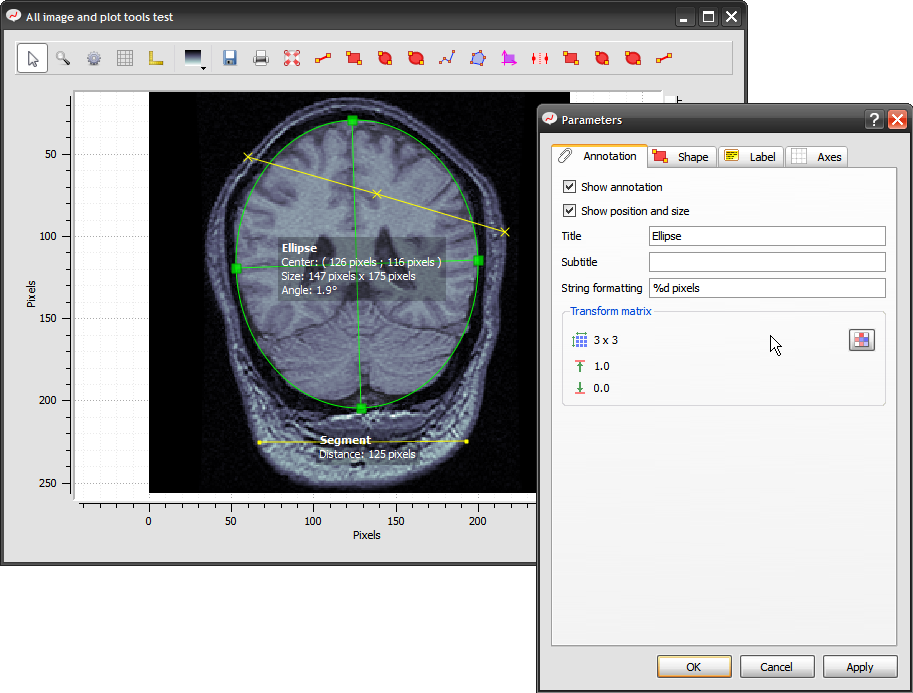
Reference¶
-
class
plotpy.tools.
RectZoomTool
(manager, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
activate
()¶ Activate tool
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
SelectTool
(manager, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ Graphical Object Selection Tool
-
activate
()¶ Activate tool
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
SelectPointTool
(manager, mode='reuse', on_active_item=False, title=None, icon=None, tip=None, end_callback=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, marker_style=None, switch_to_default_tool=None)[source]¶ -
activate
()¶ Activate tool
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
MultiLineTool
(manager, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
mouse_press
(filter, event)[source]¶ We create a new shape if it’s the first point otherwise we add a new point
-
move
(filter, event)[source]¶ moving while holding the button down lets the user position the last created point
-
activate
()¶ Activate tool
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
FreeFormTool
(manager, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
-
activate
()¶ Activate tool
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
mouse_release
(filter, event)¶ Releasing the mouse button validate the last point position
-
move
(filter, event)¶ moving while holding the button down lets the user position the last created point
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
LabelTool
(manager, handle_label_cb=None, label_style=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
activate
()¶ Activate tool
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
RectangleTool
(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
activate
()¶ Activate tool
-
add_shape_to_plot
(plot, p0, p1)¶ Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
get_shape
()¶ Reimplemented RectangularActionTool method
-
handle_final_shape
(shape)¶ To be reimplemented
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_shape
(shape)¶ To be reimplemented
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
PointTool
(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
activate
()¶ Activate tool
-
add_shape_to_plot
(plot, p0, p1)¶ Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
get_shape
()¶ Reimplemented RectangularActionTool method
-
handle_final_shape
(shape)¶ To be reimplemented
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_shape
(shape)¶ To be reimplemented
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
SegmentTool
(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
activate
()¶ Activate tool
-
add_shape_to_plot
(plot, p0, p1)¶ Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
get_shape
()¶ Reimplemented RectangularActionTool method
-
handle_final_shape
(shape)¶ To be reimplemented
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_shape
(shape)¶ To be reimplemented
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
CircleTool
(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
activate
()¶ Activate tool
-
add_shape_to_plot
(plot, p0, p1)¶ Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
get_shape
()¶ Reimplemented RectangularActionTool method
-
handle_final_shape
(shape)¶ To be reimplemented
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_shape
(shape)¶ To be reimplemented
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
EllipseTool
(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
activate
()¶ Activate tool
-
add_shape_to_plot
(plot, p0, p1)¶ Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
get_shape
()¶ Reimplemented RectangularActionTool method
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_shape
(shape)¶ To be reimplemented
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
PlaceAxesTool
(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
activate
()¶ Activate tool
-
add_shape_to_plot
(plot, p0, p1)¶ Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
get_shape
()¶ Reimplemented RectangularActionTool method
-
handle_final_shape
(shape)¶ To be reimplemented
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_shape
(shape)¶ To be reimplemented
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
AnnotatedRectangleTool
(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
activate
()¶ Activate tool
-
add_shape_to_plot
(plot, p0, p1)¶ Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
get_shape
()¶ Reimplemented RectangularActionTool method
-
handle_final_shape
(shape)¶ To be reimplemented
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_shape
(shape)¶ To be reimplemented
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
AnnotatedCircleTool
(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
activate
()¶ Activate tool
-
add_shape_to_plot
(plot, p0, p1)¶ Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
get_shape
()¶ Reimplemented RectangularActionTool method
-
handle_final_shape
(shape)¶ To be reimplemented
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_shape
(shape)¶ To be reimplemented
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
AnnotatedEllipseTool
(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
activate
()¶ Activate tool
-
add_shape_to_plot
(plot, p0, p1)¶ Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
get_shape
()¶ Reimplemented RectangularActionTool method
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_shape
(shape)¶ To be reimplemented
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
AnnotatedPointTool
(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
activate
()¶ Activate tool
-
add_shape_to_plot
(plot, p0, p1)¶ Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
get_shape
()¶ Reimplemented RectangularActionTool method
-
handle_final_shape
(shape)¶ To be reimplemented
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_shape
(shape)¶ To be reimplemented
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
AnnotatedSegmentTool
(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
activate
()¶ Activate tool
-
add_shape_to_plot
(plot, p0, p1)¶ Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
get_shape
()¶ Reimplemented RectangularActionTool method
-
handle_final_shape
(shape)¶ To be reimplemented
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_shape
(shape)¶ To be reimplemented
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
HRangeTool
(manager, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
activate
()¶ Activate tool
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
DummySeparatorTool
(manager, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>)[source]¶ -
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
AntiAliasingTool
(manager)[source]¶ -
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
-
class
plotpy.tools.
DisplayCoordsTool
(manager)[source]¶ Create and return menu for the tool’s action
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
class
plotpy.tools.
ReverseYAxisTool
(manager)[source]¶ -
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
-
class
plotpy.tools.
AspectRatioTool
(manager)[source]¶ Create and return menu for the tool’s action
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
class
plotpy.tools.
PanelTool
(manager)[source]¶ -
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
-
class
plotpy.tools.
ItemListPanelTool
(manager)[source]¶ -
activate_command
(plot, checked)¶ Activate tool
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
-
class
plotpy.tools.
ContrastPanelTool
(manager)[source]¶ -
activate_command
(plot, checked)¶ Activate tool
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
-
class
plotpy.tools.
ColormapTool
(manager, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>)[source]¶ Create and return menu for the tool’s action
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
class
plotpy.tools.
XCSPanelTool
(manager)[source]¶ -
activate_command
(plot, checked)¶ Activate tool
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
-
class
plotpy.tools.
YCSPanelTool
(manager)[source]¶ -
activate_command
(plot, checked)¶ Activate tool
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
-
class
plotpy.tools.
CrossSectionTool
(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
-
add_shape_to_plot
(plot, p0, p1)¶ Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
get_shape
()¶ Reimplemented RectangularActionTool method
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
AverageCrossSectionTool
(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]¶ -
activate
()¶ Activate tool
-
add_shape_to_plot
(plot, p0, p1)¶ Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
get_shape
()¶ Reimplemented RectangularActionTool method
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
SaveAsTool
(manager, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>)[source]¶ -
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
CopyToClipboardTool
(manager, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>)[source]¶ -
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
OpenFileTool
(manager, title='Open...', formats='*.*', toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>)[source]¶ -
SIG_OPEN_FILE
¶ Signal emitted by OpenFileTool when a file was opened (arg: filename)
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
OpenImageTool
(manager, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>)[source]¶ -
activate_command
(plot, checked)¶ Activate tool
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
SnapshotTool
(manager, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>)[source]¶ -
activate
()¶ Activate tool
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
cursor
()¶ Return tool mouse cursor
-
customEvent
(QEvent)¶
-
deactivate
()¶ Deactivate tool
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
get_shape
()¶ Reimplemented RectangularActionTool method
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
PrintTool
(manager, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>)[source]¶ -
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
SaveItemsTool
(manager, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>)[source]¶ -
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
LoadItemsTool
(manager, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>)[source]¶ -
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
AxisScaleTool
(manager)[source]¶ Create and return menu for the tool’s action
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
class
plotpy.tools.
HelpTool
(manager, toolbar_id=<class 'plotpy.tools.DefaultToolbarID'>)[source]¶ -
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
update_status
(plot)¶ called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
-
-
class
plotpy.tools.
ExportItemDataTool
(manager, toolbar_id=None)[source]¶ -
activate_command
(plot, checked)¶ Activate tool
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
-
class
plotpy.tools.
EditItemDataTool
(manager, toolbar_id=None)[source]¶ Edit item data (requires spyderlib)
-
activate_command
(plot, checked)¶ Activate tool
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
-
class
plotpy.tools.
ItemCenterTool
(manager, toolbar_id=None)[source]¶ -
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-
-
class
plotpy.tools.
DeleteItemTool
(manager, toolbar_id=None)[source]¶ -
-
blockSignals
(bool) → bool¶
-
childEvent
(QChildEvent)¶
-
children
() → list-of-QObject¶
-
connect
(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) → bool¶ QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
-
connectNotify
(SIGNAL())¶
-
create_action
(manager)¶ Create and return tool’s action
Create and return menu for the tool’s action
-
customEvent
(QEvent)¶
-
deleteLater
()¶
-
destroyed
¶ QObject.destroyed[QObject] [signal] QObject.destroyed [signal]
-
disconnect
(QObject, SIGNAL(), QObject, SLOT()) → bool¶ QObject.disconnect(QObject, SIGNAL(), callable) -> bool
-
disconnectNotify
(SIGNAL())¶
-
dumpObjectInfo
()¶
-
dumpObjectTree
()¶
-
dynamicPropertyNames
() → list-of-QByteArray¶
-
emit
(SIGNAL(), ...)¶
-
event
(QEvent) → bool¶
-
eventFilter
(QObject, QEvent) → bool¶
-
findChild
(type, str name='') → QObject¶ QObject.findChild(tuple, str name=’‘) -> QObject
-
findChildren
(type, str name='') → list-of-QObject¶ QObject.findChildren(tuple, str name=’‘) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
-
inherits
(str) → bool¶
-
installEventFilter
(QObject)¶
-
isWidgetType
() → bool¶
-
killTimer
(int)¶
-
metaObject
() → QMetaObject¶
-
moveToThread
(QThread)¶
-
objectName
() → str¶
-
parent
() → QObject¶
-
property
(str) → object¶
-
pyqtConfigure
(...)¶ Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
-
receivers
(SIGNAL()) → int¶
-
register_plot
(baseplot)¶ Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
-
removeEventFilter
(QObject)¶
-
sender
() → QObject¶
-
senderSignalIndex
() → int¶
-
setObjectName
(str)¶
-
setParent
(QObject)¶
-
setProperty
(str, object) → bool¶
-
set_parent_tool
(tool)¶ Used to organize tools automatically in menu items
-
setup_toolbar
(toolbar)¶ Setup tool’s toolbar
-
signalsBlocked
() → bool¶
-
startTimer
(int) → int¶
-
thread
() → QThread¶
-
timerEvent
(QTimerEvent)¶
-
tr
(str, str disambiguation=None, int n=-1) → str¶
-
trUtf8
(str, str disambiguation=None, int n=-1) → str¶
-