Simevent¶
The Simevent module has three classes namely: Randomsim, Simulate and Simtable. They are all used to simulate discrete events e.g. in a workplace scenario.
Randomsim¶
Randomsim helps to quickly simulate and generate a scenario with random values by just passing your desired argument values into it. It takes between 0 to 3 arguments. The generated results are then stored into the inter-arrival time and service time list needed to generate the rest of the values.
Methods¶
This class has eight methods that can be called on its instance namely:
- intarrival() —-> Displays the inter-arrival time in a list.
- arrival() —-> Displays the arrival time in a list.
- service() —-> Displays the service time in a list.
- servbegin() —-> Display the time service begins in a list.
- servend() —-> Display the time service ends in a list.
- queuewait() —-> Display the time the customer spent waiting in a list.
- custspend() —-> Display the time the customer spent in the system i.e. total time of service.
- idle() —> Display the idle time of the server (cashier).
Importing the Randomsim class¶
You can import the Randomsim class in three ways:
import eventsim.simevent
from eventsim.simevent import Randomsim
from eventsim.simevent import *
and you can then respectively use it like this:
sample = eventsim.simevent.Randomsim(6)
sample = Randomsim(6)
The following examples will cover how arguments are used in the Randomsim class. In the examples, we assume that the class has been imported first as:
from eventsim.simevent import Randomsim
Example 1a¶
zero arguments
What this does is populate the inter-arrival and service time list with random numbers (between 1 and 10) random amount of times (2 to 20). The first value of the inter-arrival time defaults to 0.
sample = Randomsim()
print("Inter-arrival time:",sample.intarrival())
print("Arrival time:",sample.arrival())
print("Service time:",sample.service())
print("Time when Service begins:",sample.servbegin())
print("Time when Service ends:",sample.servend())
print("Time customer spends waiting in Queue:",sample.queuewait())
print("Time customer spends in system:",sample.custspend())
print("Idle time of server:",sample.idle())
Result 1a¶
Inter-arrival time: [0, 8, 4, 10, 1]
Arrival time: [0, 8, 12, 22, 23]
Service time: [2, 9, 4, 5, 5]
Time when Service begins: [0, 8, 17, 22, 27]
Time when Service ends: [2, 17, 21, 27, 32]
Time customer spends waiting in Queue: [0, 0, 5, 0, 4]
Time customer spends in system: [2, 9, 9, 5, 9]
Idle time of server: [0, 6, 0, 1, 0]
Example 1b¶
one argument
Usage: Randomsim(list size)
What this does is populate the inter-arrival and service time list with random numbers (between 1 and 10) the amount of times supplied as the only argument value. The first value of the inter-arrival time defaults to 0.
sample = Randomsim(6)
print("Inter-arrival time:",sample.intarrival())
print("Arrival time:",sample.arrival())
print("Service time:",sample.service())
print("Time when Service begins:",sample.servbegin())
print("Time when Service ends:",sample.servend())
print("Time customer spends waiting in Queue:",sample.queuewait())
print("Time customer spends in system:",sample.custspend())
print("Idle time of server:",sample.idle())
Result 1b¶
Inter-arrival time: [0, 4, 10, 9, 1, 9]
Arrival time: [0, 4, 14, 23, 24, 33]
Service time: [4, 10, 1, 8, 10, 5]
Time when Service begins: [0, 4, 14, 23, 31, 41]
Time when Service ends: [4, 14, 15, 31, 41, 46]
Time customer spends waiting in Queue: [0, 0, 0, 0, 7, 8]
Time customer spends in system: [4, 10, 1, 8, 17, 13]
Idle time of server: [0, 0, 0, 8, 0, 0]
Example 1c¶
two arguments
Usage: Randomsim(max value, list size)
What this does is populate the inter-arrival and service time list with numbers not more than the first argument starting from 1. The list is populated the amount of times specified as the second argument.
sample = Randomsim(4,6)
print("Inter-arrival time:",sample.intarrival())
print("Arrival time:",sample.arrival())
print("Service time:",sample.service())
print("Time when Service begins:",sample.servbegin())
print("Time when Service ends:",sample.servend())
print("Time customer spends waiting in Queue:",sample.queuewait())
print("Time customer spends in system:",sample.custspend())
Result 1c¶
Inter-arrival time: [0, 1, 2, 2, 2, 4]
Arrival time: [0, 1, 3, 5, 7, 11]
Service time: [1, 2, 1, 4, 2, 4]
Time when Service begins: [0, 1, 3, 5, 9, 11]
Time when Service ends: [1, 3, 4, 9, 11, 15]
Time customer spends waiting in Queue: [0, 0, 0, 0, 2, 0]
Time customer spends in system: [1, 2, 1, 4, 4, 4]
Idle time of server: [0, 0, 0, 1, 0, 0]
Example 1d¶
three arguments
Usage: Randomsim(max value for inter-arrival time, max value for service time, list size)
What this does is populate the inter-arrival with numbers between 1 and the value passed into the first argument, service time is populated with values between 1 and the second argument while the third argument is the amount/size of the two lists.
sample = Randomsim(4, 6, 8)
print("Inter-arrival time:",sample.intarrival())
print("Arrival time:",sample.arrival())
print("Service time:",sample.service())
print("Time when Service begins:",sample.servbegin())
print("Time when Service ends:",sample.servend())
print("Time customer spends waiting in Queue:",sample.queuewait())
print("Time customer spends in system:",sample.custspend())
Result 1d¶
Inter-arrival time: [0, 3, 2, 2, 3, 3, 3, 4]
Arrival time: [0, 3, 5, 7, 10, 13, 16, 20]
Service time: [6, 2, 2, 2, 4, 5, 6, 4]
Time when Service begins: [0, 6, 8, 10, 12, 16, 21, 27]
Time when Service ends: [6, 8, 10, 12, 16, 21, 27, 31]
Time customer spends waiting in Queue: [0, 3, 3, 3, 2, 3, 5, 7]
Time customer spends in system: [6, 5, 5, 5, 6, 8, 11, 11]
Idle time of server: [0, 0, 0, 0, 0, 0, 0, 0]
Simulate¶
Simulate helps to quickly simulate and generate a scenario with user-defined values. It is more flexible in that it allows you to input your own data rather than input just numbers as compared to Randomsim. It can take one or two arguments which are inter-arrival time list and service time list. They must be of the same length.
Methods¶
This class has eight methods (same as Randomsim) that can be called on its instance namely:
- intarrival() —-> Displays the inter-arrival time in a list.
- arrival() —-> Displays the arrival time in a list.
- service() —-> Displays the service time in a list.
- servbegin() —-> Display the time service begins in a list.
- servend() —-> Display the time service ends in a list.
- queuewait() —-> Display the time the customer spent waiting in a list.
- custspend() —-> Display the time the customer spent in the system i.e. total time of service.
- idle() —> Display the idle time of the server (cashier).
Importing the Simulate class¶
You can import the Simulate class in three ways:
import eventsim.simevent
from eventsim.simevent import Simulate
from eventsim.simevent import *
and you can then respectively use it like this:
sample = eventsim.simevent.Simulate([5, 6, 3], [4,7,2])
sample = Simulate([7, 9, 6])
The following examples will cover how arguments are used in the Simulate class. In the examples, we assume that the class has been imported first as:
from eventsim.simevent import Simulate
Example 2a¶
one argument
Usage: Simulate(inter-arrival list)
What this does is populate the inter-arrival time with the user-defined argument (must be a list) and service time would be automatically generated (populated with numbers between 1 and 10 with the same size as inter-arrival time).
sample = Simulate([7, 9, 6])
print("Inter-arrival time:",sample.intarrival())
print("Arrival time:",sample.arrival())
print("Service time:",sample.service())
print("Time when Service begins:",sample.servbegin())
print("Time when Service ends:",sample.servend())
print("Time customer spends waiting in Queue:",sample.queuewait())
print("Time customer spends in system:",sample.custspend())
print("Idle time of server:",sample.idle())
Result 2a¶
Inter-arrival time: [7, 9, 6]
Arrival time: [7, 16, 22]
Service time: [2, 5, 1]
Time when Service begins: [7, 16, 22]
Time when Service ends: [9, 21, 23]
Time customer spends waiting in Queue: [0, 0, 0]
Time customer spends in system: [2, 5, 1]
Idle time of server: [0, 7, 1]
Example 2b¶
two arguments
Usage: Randomsim(inter-arrival list, service time list)
What this does is populate the inter-arrival with the first list argument and the service time with the second argument which is then used to calcuate the the rest.
sample = Simulate([0, 5, 6, 3, 7, 9, 3], [4, 7, 2, 1, 3, 11, 7])
print("Inter-arrival time:",sample.intarrival())
print("Arrival time:",sample.arrival())
print("Service time:",sample.service())
print("Time when Service begins:",sample.servbegin())
print("Time when Service ends:",sample.servend())
print("Time customer spends waiting in Queue:",sample.queuewait())
print("Time customer spends in system:",sample.custspend())
print("Idle time of server:",sample.idle())
Result 2b¶
Inter-arrival time: [0, 5, 6, 3, 7, 9, 3]
Arrival time: [0, 5, 11, 14, 21, 30, 33]
Service time: [4, 7, 2, 1, 3, 11, 7]
Time when Service begins: [0, 5, 12, 14, 21, 30, 41]
Time when Service ends: [4, 12, 14, 15, 24, 41, 48]
Time customer spends waiting in Queue: [0, 0, 1, 0, 0, 0, 8]
Time customer spends in system: [4, 7, 3, 1, 3, 11, 15]
Idle time of server: [0, 1, 0, 0, 6, 6, 0]
Simtable¶
Simtable is used to display generated results in a tabled format using the tkinter module.
Note
First of all make sure you have tkinter or Tkinter installed (Mostly always comes with your python distribution). It is advisable to use the import * statement if you want to use the Simtable class because of the difference in the old and new tkinter module. If you used the import * mentioned previously, the program will try to fix this problem for you. Otherwise, make sure you have imported tkinter or Tkinter (whichever works). or use this:
from eventsim.simevent import *
this should be sufficient to use the table but remember you also need to create an instance of your generated outcome from the Randomsim or Simulate class which can then be passed as the first argument of Simtable.
But if you aren’t satisfied with the above code or you still get an error, try this:
from eventsim.simevent import Simtable #Import any other class you want to use here (either Randomsim or simulate)try: from tkinter import * #for python 3 except ImportError: from Tkinter import * # for from ttk import * # python 2
Methods¶
This class has only one method drawtable() for representing data in a table.
Importing the Simtable class¶
You can import the Simtable class in three ways but the third one is the recommended method. That way importing the tkinter module is not really needed:
import eventsim.simevent
from eventsim.simevent import Simtable
from eventsim.simevent import *
and you can then respectively use it like this:
sampletable = eventsim.simevent.Simtable(sample, Tk())
sampletable = Simtable(sample, Tk())
The following example will cover how arguments are used in the Simulate class. In the example, we assume that the class has been imported first as:
from eventsim.simevent import *
Example 3¶
Usage: Simtable(classInstance, parent)
where parent must always be Tk()
sample = Simulate([0, 5, 6, 3, 7, 9, 3], [4, 7, 2, 1, 3, 11, 7])
sampletable = Simtable(sample, Tk())
sampletable.drawtable()
Result¶
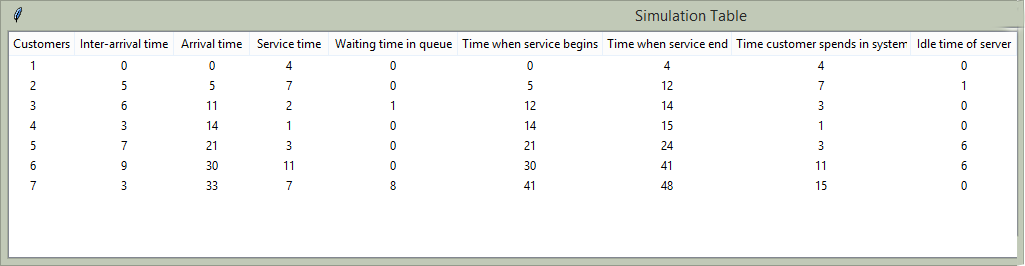
Note
Just like the above eample, you can also use it on both the Randomsim and Simulate class instance the same way. sampletable is just an instance of the Simtable and can be any values. In brief, sampletable is an instance of Simtable that takes an instance of any other simevent class as the first argument and Tk() as the second argument.