PLLphaseNoise¶
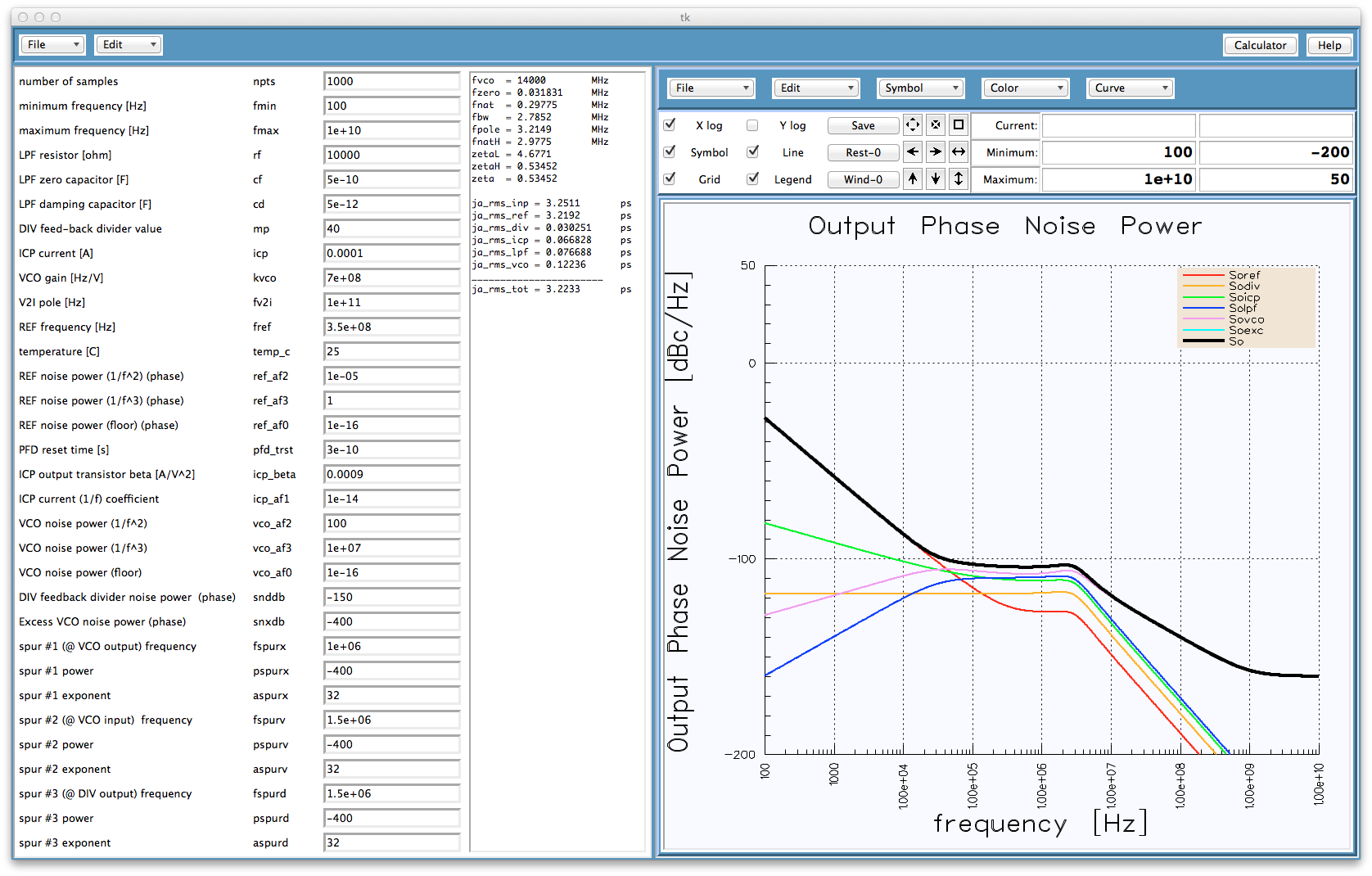
-
class
decida.PLLphaseNoise.
PLLphaseNoise
(parent=None, **kwargs)¶ Bases:
decida.ItclObjectx.ItclObjectx
,Tkinter.Frame
synopsis:
Phase-Locked Loop phase noise analysis.
PLLphaseNoise calculates the frequency-domain noise power spectra of a PLL. The different noise components within the PLL are specified by different models for the noise, and each component’s effect on the output of the PLL is calculated by the respective transfer-function from the place within the PLL to the output.
PLLphaseNoise has two modes of use. The first is an interactive mode, with a graphical user-interface. In the interactive mode, the user changes an input PLL parameter value, or noise model parameter in the entry box and presses <Return> to cause a new set of calculations to be performed and displayed.
In the non-interactive mode, PLLphaseNoise can be embedded in a script involving one or more loops of calculations. In this mode, use the recalculate user method. After re-calculation, PLL and phase-noise metrics can be reported to a file.
The DeCiDa application pll_phase_noise simply instantiates one PLLphaseNoise object.
constructor arguments:
parent (Tkinter handle, default=None)
handle of frame or other widget to pack PLL in. if this is not specified, top-level is created.**kwargs (dict)
keyword=value specifications: configuration-optionsconfiguration options:
verbose (bool, default=False)
Enable/disable verbose mode.gui (bool, default=True)
If gui is True, display interactive graphical-user interface. Otherwise, perform the calculations without any user interaction.plot_width (str, default=”5in”)
Width of plot window (Tk inch or pixel specification)plot_height (str, default=”5in”)
Height of plot window (Tk inch or pixel specification)plot_style (int, default=0)
The style of the plot:
- 0 : plot Output Noise Power
- 1 : plot Noise Sources
- 2 : plot Transfer Functions
plot_orient (str, default=”horizontal”)
One of “horizontal” or “vertical”. If horizontol, parameter selection and plot panes are side-by-side. If vertical, parameter selection pane is over plot pane.plot_title (str, default=””)
Main plot titlenpts (int, default=1000)
Number of frequency points to sample (logarithmic sampliing).fmax (float, default=1e10)
The maximum frequency to sample.fmin (float, default=1e2)
The minimum frequency to sample.rf (float, default=10e3)
Loop filter resistor value [ohm]cf (float, default=500e_12)
Loop filter capacitor value [F]cd (float, default=5e_12)
Loop filter ripple-bypass capacitor value [F]mp (float, default=40.0)
Feed-back divider value.icp (float, default=100e_6)
Charge-pump current value. [A]kvco (float, default=700e6)
VCO total gain. [Hz/V]fv2i (float, default=100e9)
Voltage to current converter pole. [Hz]fref (float, default=350e6)
Reference frequency. [Hz]temp_c (float, default=25.0)
Circuit temperature. [C]ref_af2 (float, default=1e_5)
REF (1/freq^2) noise power. [rad^2*Hz]ref_af3 (float, default=1)
REF (1/freq^3) noise power. [rad^2*Hz^2]ref_af0 (float, default=1e_16)
REF noise power floor. [rad^2/Hz]pfd_trst (float, default=300e_12)
Phase-Frequency Detector reset time. [s]icp_beta (float, default=900e_6)
Charge-pump output transistor transconductance parameter. [A/V^2]icp_af1 (float, default=1e_14)
Charge-pump current 1/f coefficient. [A^2]vco_af2 (float, default=100.0)
VCO (1/freq^2) noise power. [rad^2*Hz]vco_af3 (float, default=1e7)
VCO (1/freq^3) noise power. [rad^2*Hz^2]vco_af0 (float, default=1e_16)
VCO noise power floor. [rad^2/Hz]snddb (float, default=_150.0)
Feedback divider noise power. [rad^2/Hz]snxdb (float, default=_400.0)
Excess VCO noise power. [rad^2/Hz]fspurx (float, default=1.0e6)
Noise spur 1 (at VCO output) frequency. [Hz]pspurx (float, default=_400.0)
Noise spur 1 (at VCO output) power. [rad^2/Hz]aspurx (float, default=32)
Noise spur 1 (at VCO output) exponent.fspurv (float, default=1.5e6)
Noise spur 2 (at VCO input) frequency. [Hz]pspurv (float, default=_400.0)
Noise spur 2 (at VCO input) power. [V^2/Hz]aspurv (float, default=32)
Noise spur 2 (at VCO input) exponent.fspurd (float, default=1.5e6)
Noise spur 3 (at divider output) frequency. [Hz]pspurd (float, default=_400.0)
Noise spur 3 (at divider output) power. [rad^2/Hz]aspurd (float, default=32)
Noise spur 3 (at divider output) exponent.example (from test_PLLphaseNoise):
from decida.PLLphaseNoise import PLLphaseNoise PLLphaseNoise()
public methods:
- public methods from ItclObjectx
-
get
(par)¶ get a PLLphaseNoise parameter or metric.
arguments:
par (str)
PLLphaseNoise configuration option or one of the following metrics:
- lpll : PLL inductance, mp/(Kvco*Icp)
- zo : PLL characteristic impedance, sqrt(lpll/cf)
- zeta : PLL damping coefficient
- Q : PLL quality factor
- wn : PLL natural frequency [rad/s]
- wb : PLL bandwidth [rad/s]
- wz : PLL zero frequency [rad/s]
- wp : PLL peak frequency [rad/s]
- wh : PLL high zeta frequency [rad/s]
- wv : PLL V to I converter frequency [rad/s]
- peak : PLL peak closed-loop jitter transfer value [dB]
- zeth : PLL high-frequency damping factor
- fn : PLL natural frequency [Hz]
- fb : PLL bandwidth [Hz]
- fz : PLL zero frequency [Hz]
- fp : PLL peak frequency [Hz]
- fh : PLL high frequency [Hz]
- fvco : VCO frequency [Hz]
- ja : Total absolute jitter at PLL output [s]
- ja_inp : Absolute jitter at PLL input reference noise [s]
- ja_ref : Absolute jitter at PLL output from reference noise [s]
- ja_div : Absolute jitter at PLL output from divider noise [s]
- ja_icp : Absolute jitter at PLL output from charge-pump noise [s]
- ja_lpf : Absolute jitter at PLL output from loop-filter noise [s]
- ja_vco : Absolute jitter at PLL output from VCO noise [s]
-
plot
(new=False)¶
-
recalculate
()¶ recalculate transfer functions, and output phase-noise.
results:
- Frequency domain transfer functions are re-calculated, noise models are updated, and output phase-noise per component is calculated and displayed. For non-gui applications, several metrics are also calculated.
-
report
(style=1)¶ return a formated PLL parameters report.
arguments:
style (int, default=1)
The style of the report to generate:
- verbose
- brief
results:
- Returns a formatted PLL report with various parameters and metrics.
-
write_data
(file=None)¶ write the calculated data to a file.
arguments:
file (str, default=None)
Specify the file to write. If not specified, use file dialog to specify the file.results:
- Writes space-separated value format file with the calculated input and output noise and transfer functions.
-
write_plot
(file=None)¶ write a PostScript plot to a file.
arguments:
file (str, default=None)
Specify the file to write. If not specified, use file dialog to specify the file.results:
- Writes a PostScript file with the current plot.
-
write_report
(file=None)¶ write PLLphaseNoise report to a file.
arguments:
file (str, default=None)
Specify the file to write. If not specified, use file dialog to specify the file.results:
- Writes a formatted PLLphaseNoise report to the file.
-
write_script
(file=None)¶ write an executable PLLphaseNoise script with the current parameter set.
arguments:
file (str, default=None)
Specify the file to write. If not specified, use file dialog to specify the file.results:
- Writes executable script, which when run displays the PLLphaseNoise output noise and transfer functions for the last set of PLLphaseNoise parameters.