Welcome to PyJones’s documentation!¶
The Jones calculus is a very convinient form for calculating the polarization of light under the influence of polarization changing optical elements. Therefore it is possible to model a whole beampath and find out the final polarization after multiple optical elements. PyJones is ment as an easy interface for these calculations in Python and prvides several predefined polarizations and optical elements which are commonly used.
The best way to understand how PyJones works is by just going through the examples and see the API. Afterwards it is advisable to have a look at the class and module documentation below so you can find out which predefined elements exist and how to use them.
Examples:¶
The following code snippet shows a classic example of the surprising effects of light polarization
In [1]: from pyjones.polarizations import LinearHorizontal
In [2]: from pyjones.opticalelements import Polarizer, PolarizerVertical
In [3]: input_polarization = LinearHorizontal()
In [4]: output_polarization = PolarizerVertical()*input_polarization
In [5]: print("Output: %s, Intensity: %.2f" %(output_polarization, output_polarization.intensity))
Output: JonesVector([0j, 0j]), Intensity: 0.00
That means the light is perfectly blocked. We now put in one more polarizer:
In [6]: output_polarization = PolarizerVertical()*Polarizer(45)*input_polarization
In [7]: print("Output: %s, Intensity: %.2f" %(output_polarization, output_polarization.intensity))
Output: JonesVector([0j, (0.5+0j)]), Intensity: 0.25
That means if we put in one more polarizer in the middle suddenly there is light again.
You can also use PyJones to visualize for example Malus’ law that states the the transmitted intensity of a linear polarized beam trough a polarizer is given by

where is the angle between the input polarization and the polarizer.
In [1]: import matplotlib.pyplot as plt
In [2]: import numpy as np
In [3]: from pyjones.polarizations import LinearHorizontal
In [4]: from pyjones.opticalelements import Polarizer
In [5]: angles = np.linspace(0, 360, 1000)
In [6]: intensities = [(Polarizer(angle)*LinearHorizontal()).intensity for angle in angles]
In [7]: plt.plot(angles, intensities);
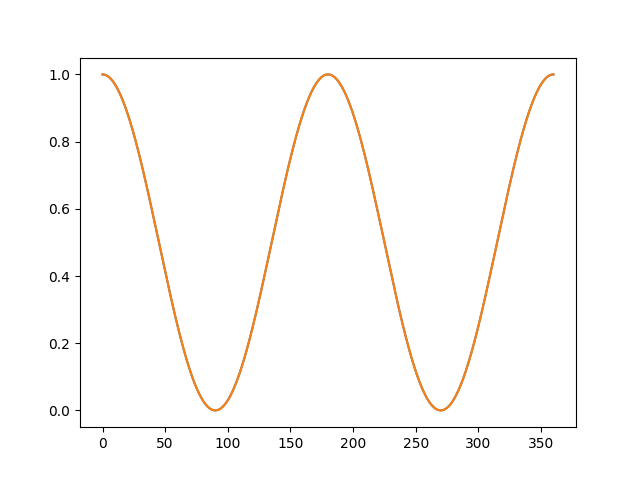
Jones Vector¶
This module provides Jones vectors as a two dimensional representation of the polarization of light. The basic class is JonesVector from which all predefined polarizations inherit from. Predefined polarizations are:
- LinearHorizontal
- LinearVertical
- LinearDiagonal
- LinearAntiDiagonal
- Linear(angle)
- CircularRight
- CircularLeft
Base Class
-
class
pyjones.polarizations.
JonesVector
(polarization, normalize=True)¶ This represents a Jones vector and is one representation of the polarisation of light.
Parameters: polarization – An two element iterable with complex numbers representing the value and phase of the Ex and Ey light component -
intensity
¶ Property which returns the intensity of the Jones vector
Returns: The intensity of the Jones vector Return type: float
-
Predefined Polarizations
-
class
pyjones.polarizations.
LinearHorizontal
¶ This is a subclass of JonesVector corresponding to linear horizontal polarisation
-
class
pyjones.polarizations.
LinearVertical
¶ This is a subclass of JonesVector corresponding to linear vertical polarisation
-
class
pyjones.polarizations.
Linear
(angle)¶ This is a subclass of JonesVector corresponding to a linear polarization with angle
Parameters: angle – Angle of the linear polarization with respect to horizontal plane
-
class
pyjones.polarizations.
LinearDiagonal
¶ This is a subclass of JonesVector corresponding to linear diagonal polarisation
-
class
pyjones.polarizations.
LinearAntidiagonal
¶ This is a subclass of JonesVector corresponding to linear antidiagonal polarisation
-
class
pyjones.polarizations.
CircularRight
¶ This is a subclass of JonesVector corresponding to right circular polarisation
-
class
pyjones.polarizations.
CircularLeft
¶ This is a subclass of JonesVector corresponding to left circular polarisation
Jones Matrix¶
This module provides optical elements which influence the polarization of light. The basic class is JonesMatrix from which all predefined optical elements inherit from. Predefined optical elements are:
- PolarizerHorizontal
- PolarizerVertical
- Polarizer(angle)
- QuarterWavePlate(angle)
- HalfWavePlate(angle)
Base Class
-
class
pyjones.opticalelements.
JonesMatrix
(matrix)¶ This is the baseclass which describes a polarization influencing optical element.
Parameters: matrix – A 2x2 matrix either of type np.matrix or a list of two two-element lists describing the effect of that optical element to the polarization state of passing light.
Predefined Optical Elements
-
class
pyjones.opticalelements.
PolarizerHorizontal
¶ This is a subclass of JonesMatrix corresponding to a horizontal polarizer
-
class
pyjones.opticalelements.
PolarizerVertical
¶ This is a subclass of JonesMatrix corresponding to a vertical polarizer
-
class
pyjones.opticalelements.
Polarizer
(angle)¶ This is a subclass of JonesMatrix corresponding to a polarizer with angle
Parameters: angle – Angle of the polarizer with respect to horizontal plane
-
class
pyjones.opticalelements.
QuarterWavePlate
(angle)¶ This is a subclass of JonesMatrix corresponding to a quarter wave plate with angle
Parameters: angle – Angle of the fast axis of the quarter wave plate with respect to horizontal plane
-
class
pyjones.opticalelements.
HalfWavePlate
(angle)¶ This is a subclass of JonesMatrix corresponding to a half wave plate with angle
Parameters: angle – Angle of the fast axis of the half wave plate with respect to horizontal plane